Galleries
Overview
Gallery is a set on photos in a grid-like design. Based on your needs, AstroPine provides you 3 types of gallery.
Discover each one and their variants with examples below.
Rest Gallery
Take RestGallery as a way to reduce display all photos. Because too many images can slower your page loading, itโs pragmatic to display a few.
With RestGallery, you set a number of images to display, and then, choose to display the others.
By default, 3 images are displayed.
P.S: Notice that itโs up to you to design gallery layout with class props. Thanks to tailwind, you can fully customize it.
Here below some examples with different displayed images.
- +
- +
- +
Copied !
---
import RestGallery from "$components/gallery/rest/RestGallery.astro";
import type { ImageType } from "$common/types";
const images: ImageType[] = [
{
src: "https://cdn.devdojo.com/images/june2023/mountains-01.jpeg",
alt: "Photo of Mountains",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-02.jpeg",
alt: "Photo of Mountains 02",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-03.jpeg",
alt: "Photo of Mountains 03",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-04.jpeg",
alt: "Photo of Mountains 04",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-05.jpeg",
alt: "Photo of Mountains 05",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-06.jpeg",
alt: "Photo of Mountains 06",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-07.jpeg",
alt: "Photo of Mountains 07",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-08.jpeg",
alt: "Photo of Mountains 08",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-09.jpeg",
alt: "Photo of Mountains 09",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-10.jpeg",
alt: "Photo of Mountains 10",
},
];
---
<div class="flex flex-col gap-y-12">
<RestGallery class="flex flex-wrap [&_li]:w-1/2" {images} />
<hr />
<RestGallery class="flex flex-wrap [&_li]:w-1/4" {images} />
<hr />
<RestGallery
nbDisplayedImages={4}
class="grid grid-cols-2 w-3/4 mx-auto gap-2"
{images}
/>
</div>
Carousel Gallery
A trendy gallery is obviously carousel. Itโs a combination of Carousel component with a set of thumbnails as indicators.
You can change direction with direction props and define the thumbnail width with thumbnailWidth props.
See example below:
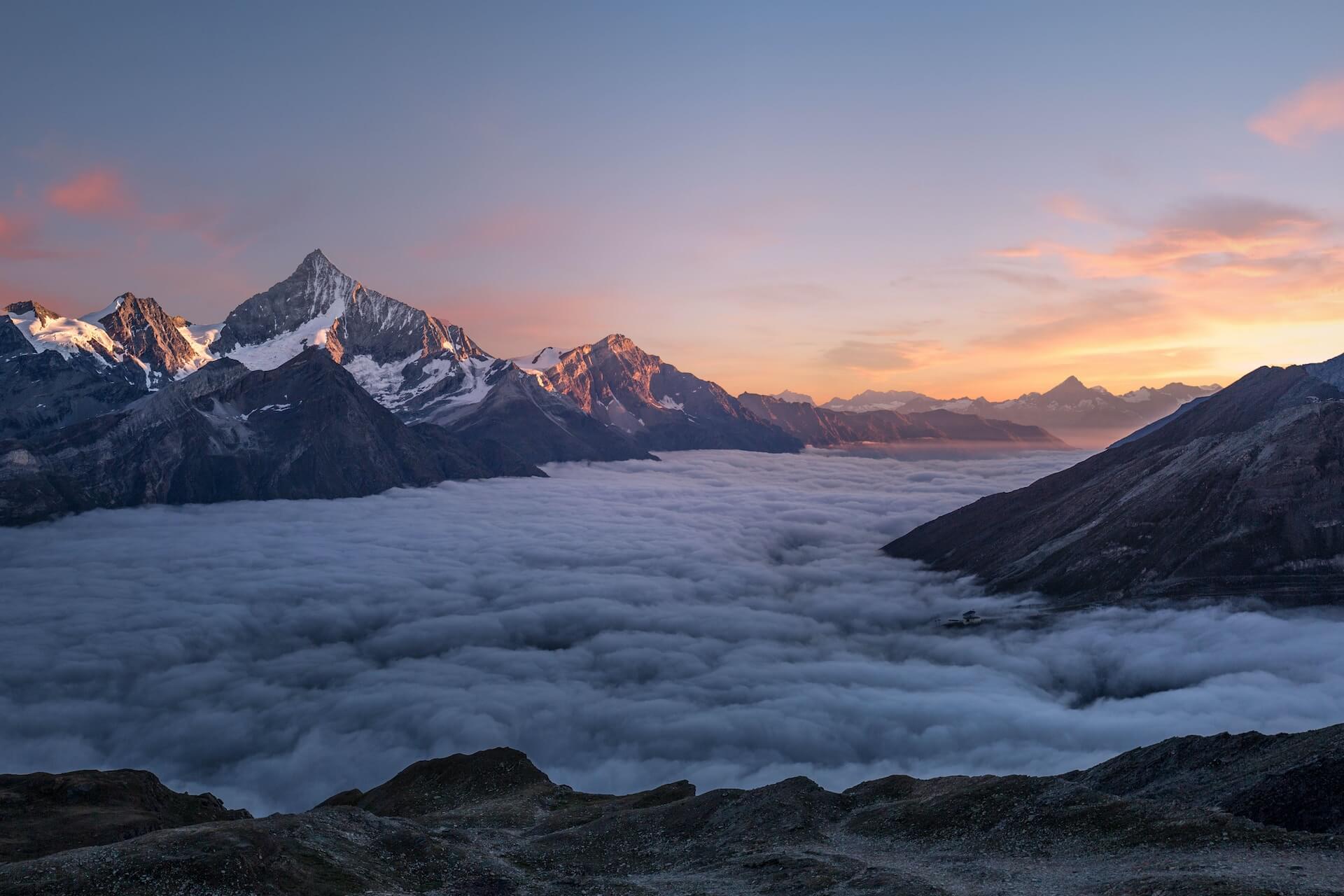
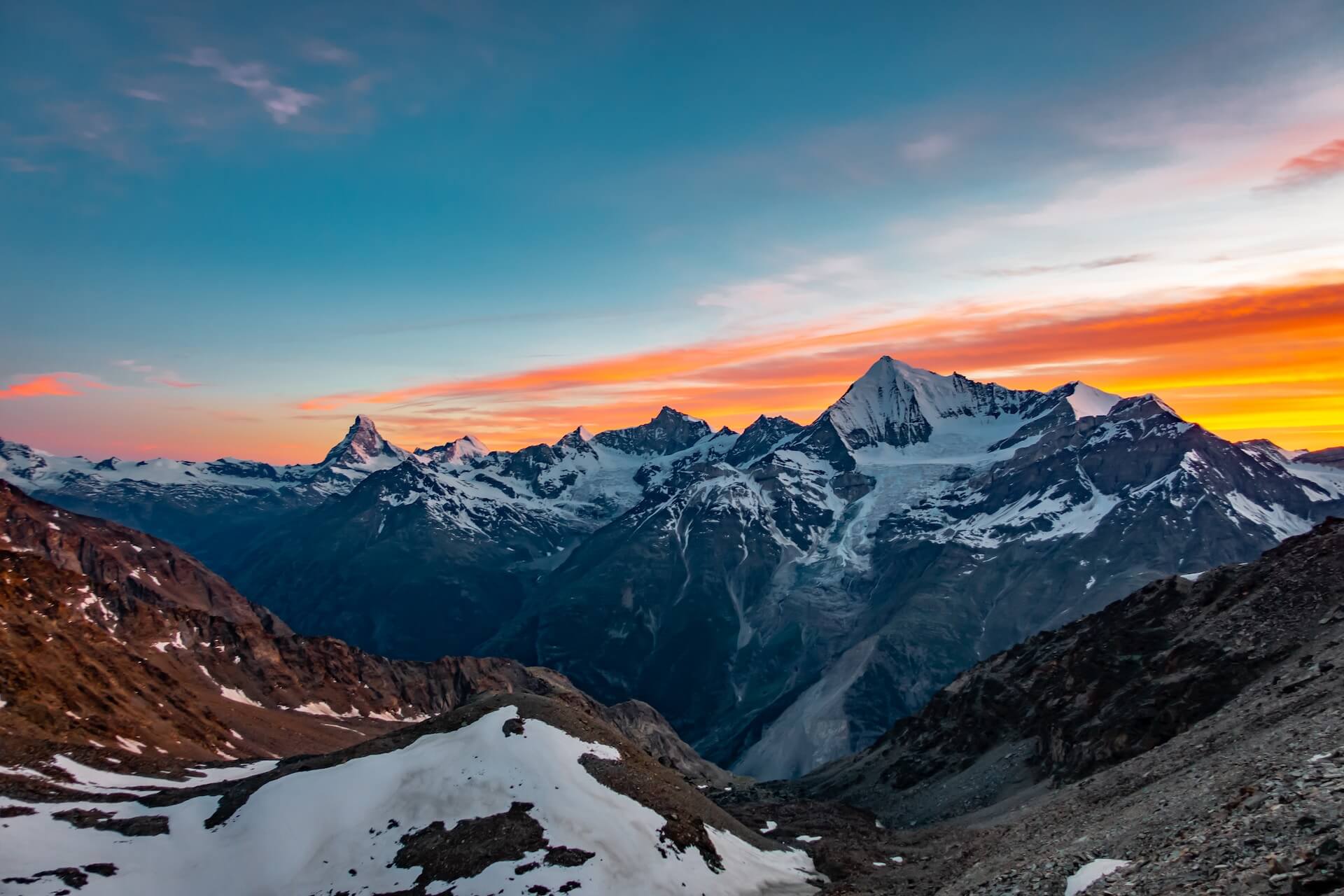
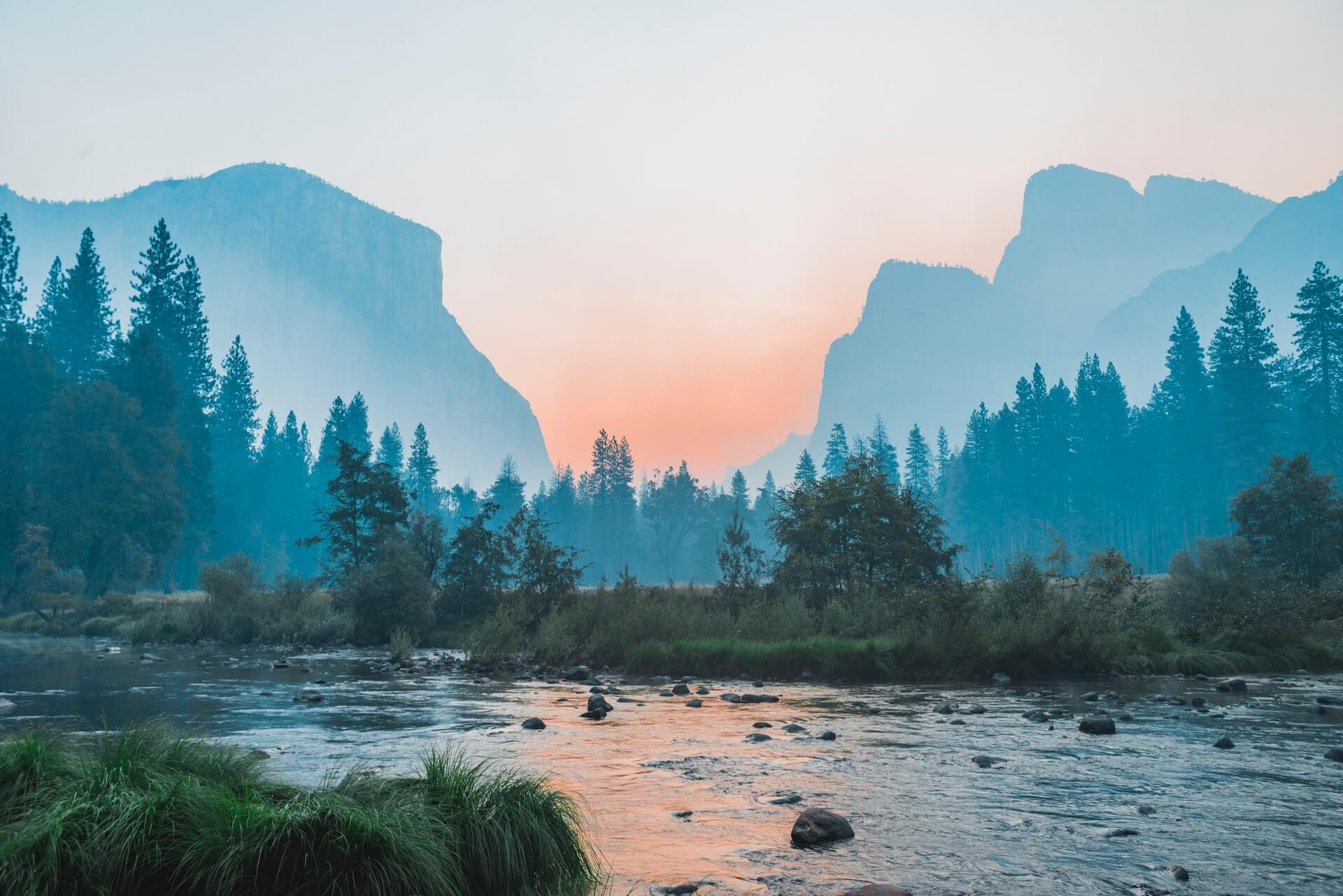
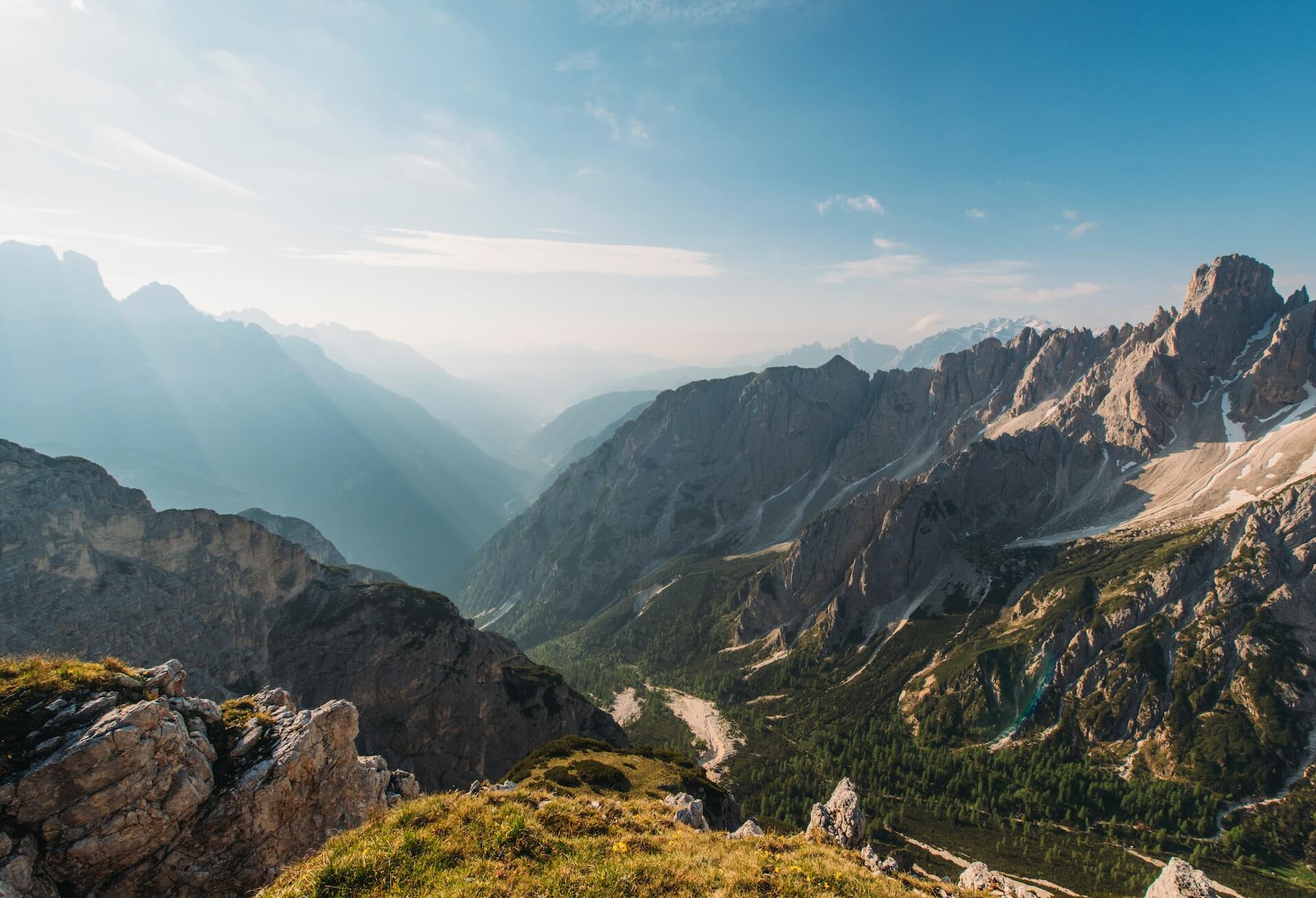
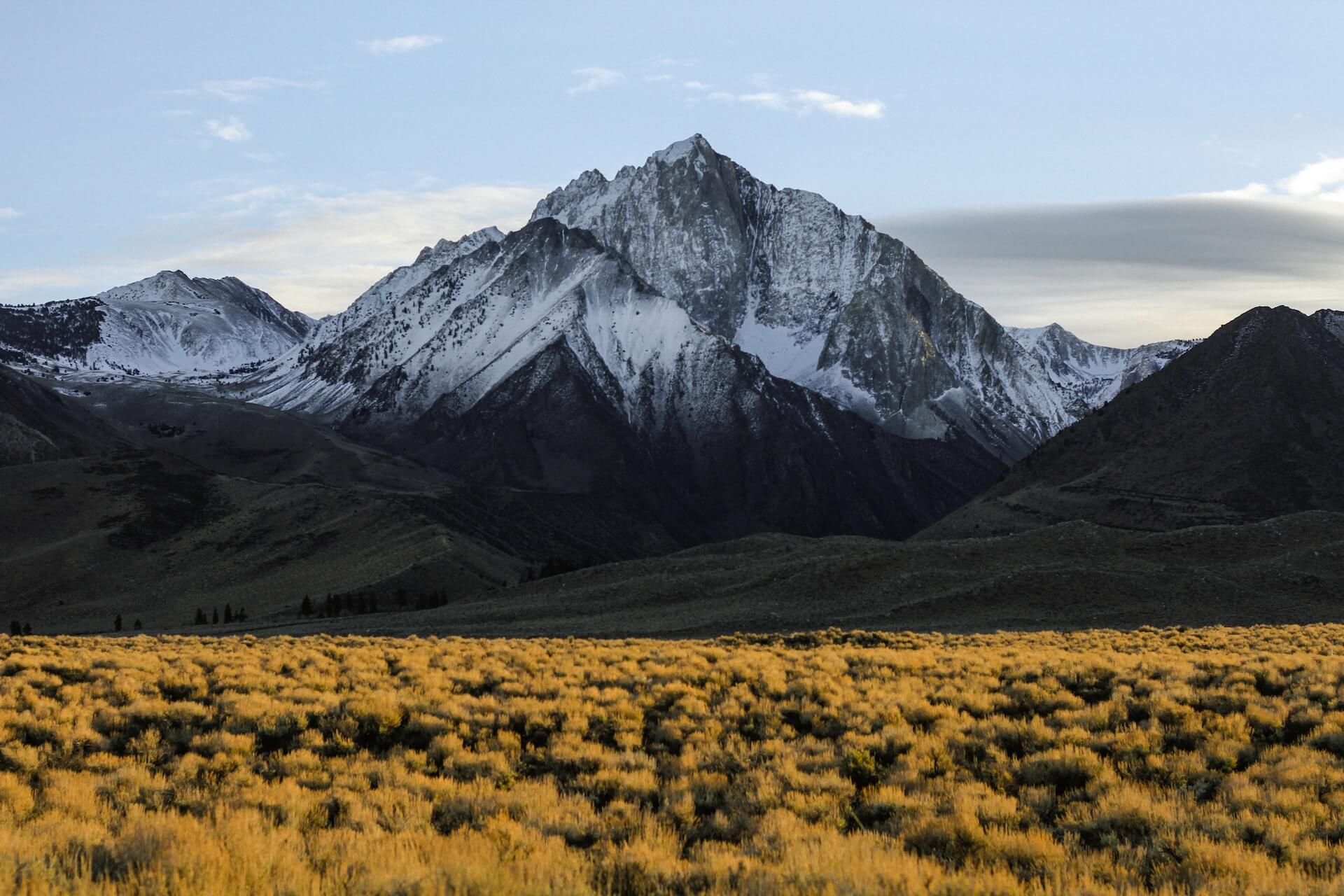
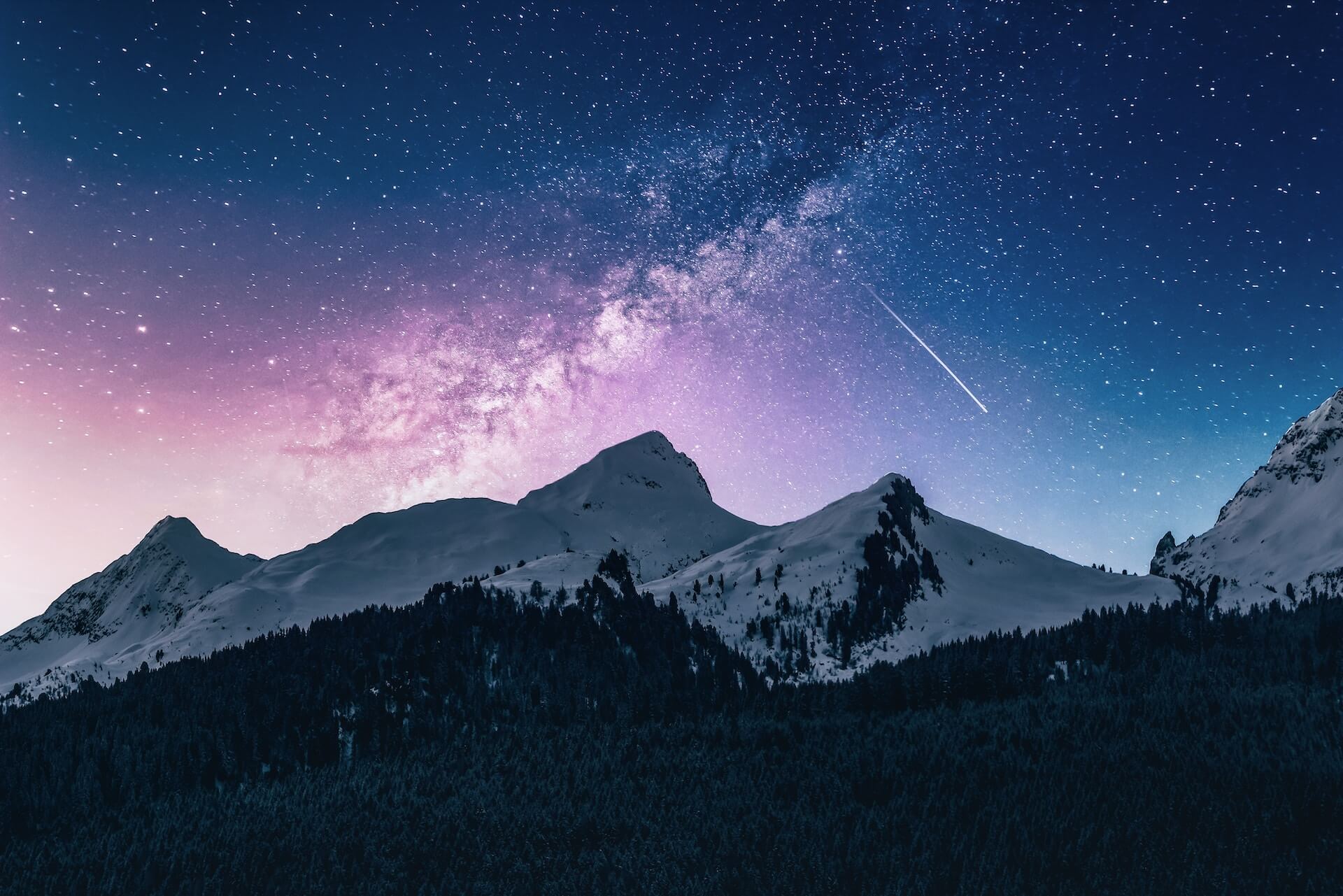
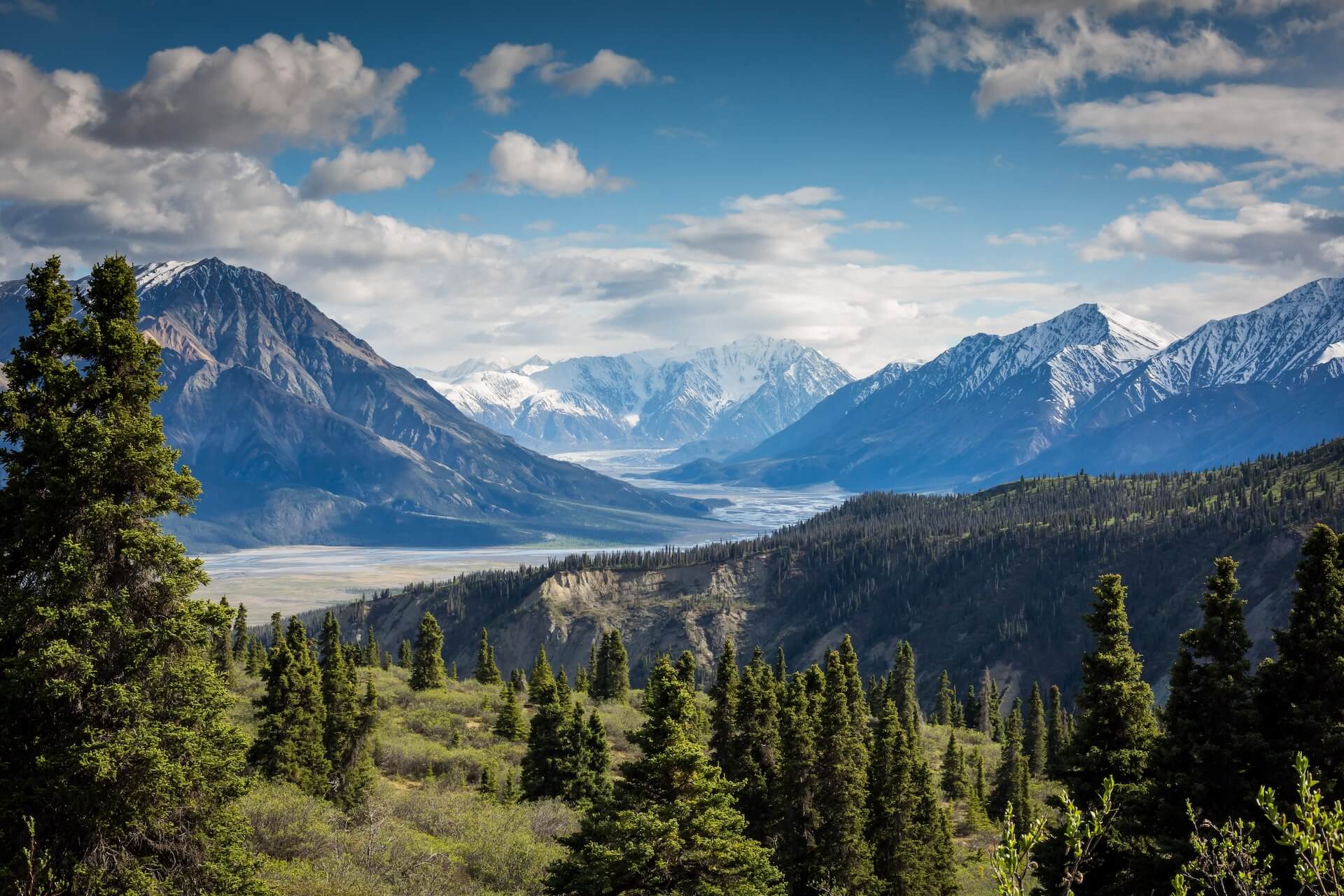
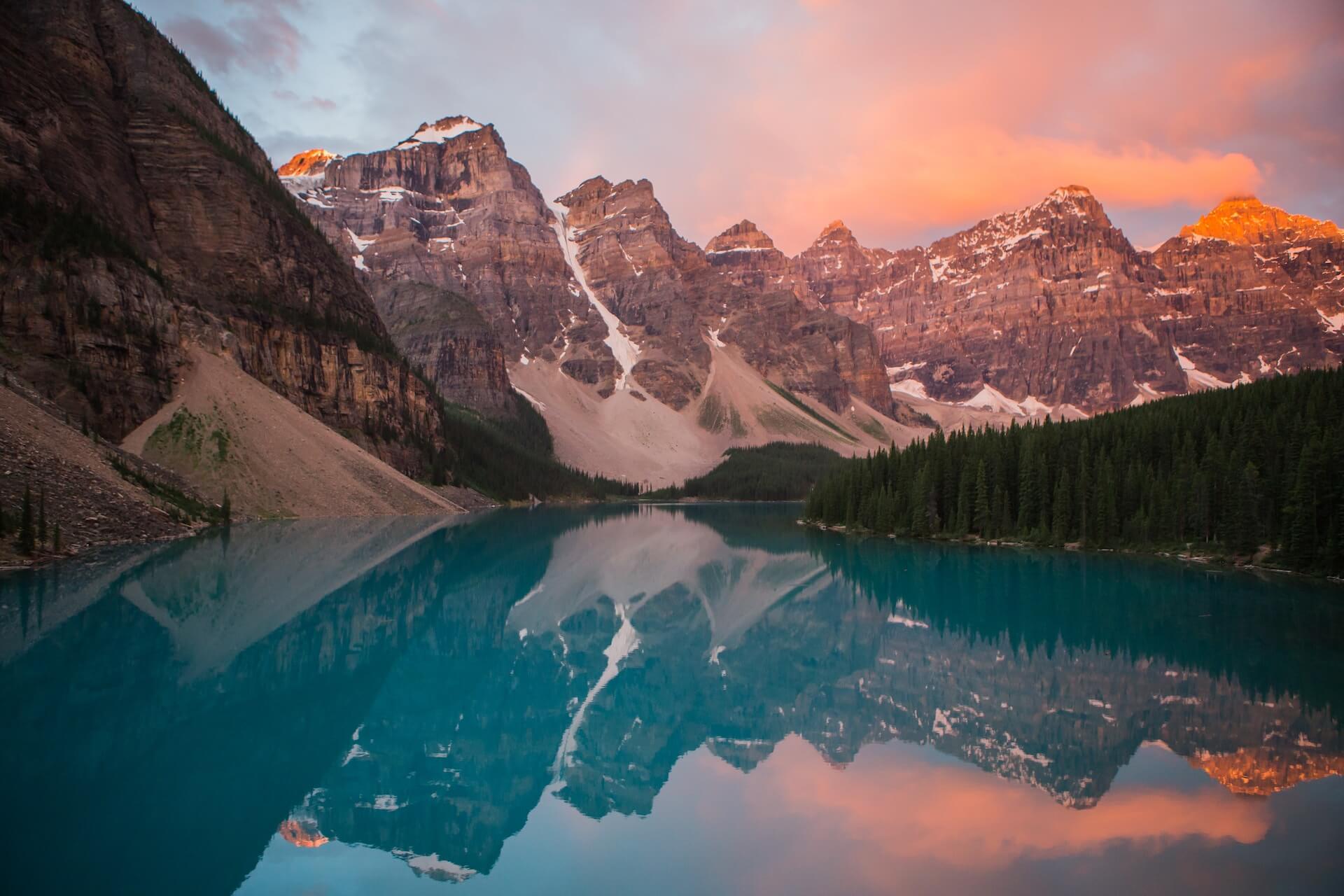
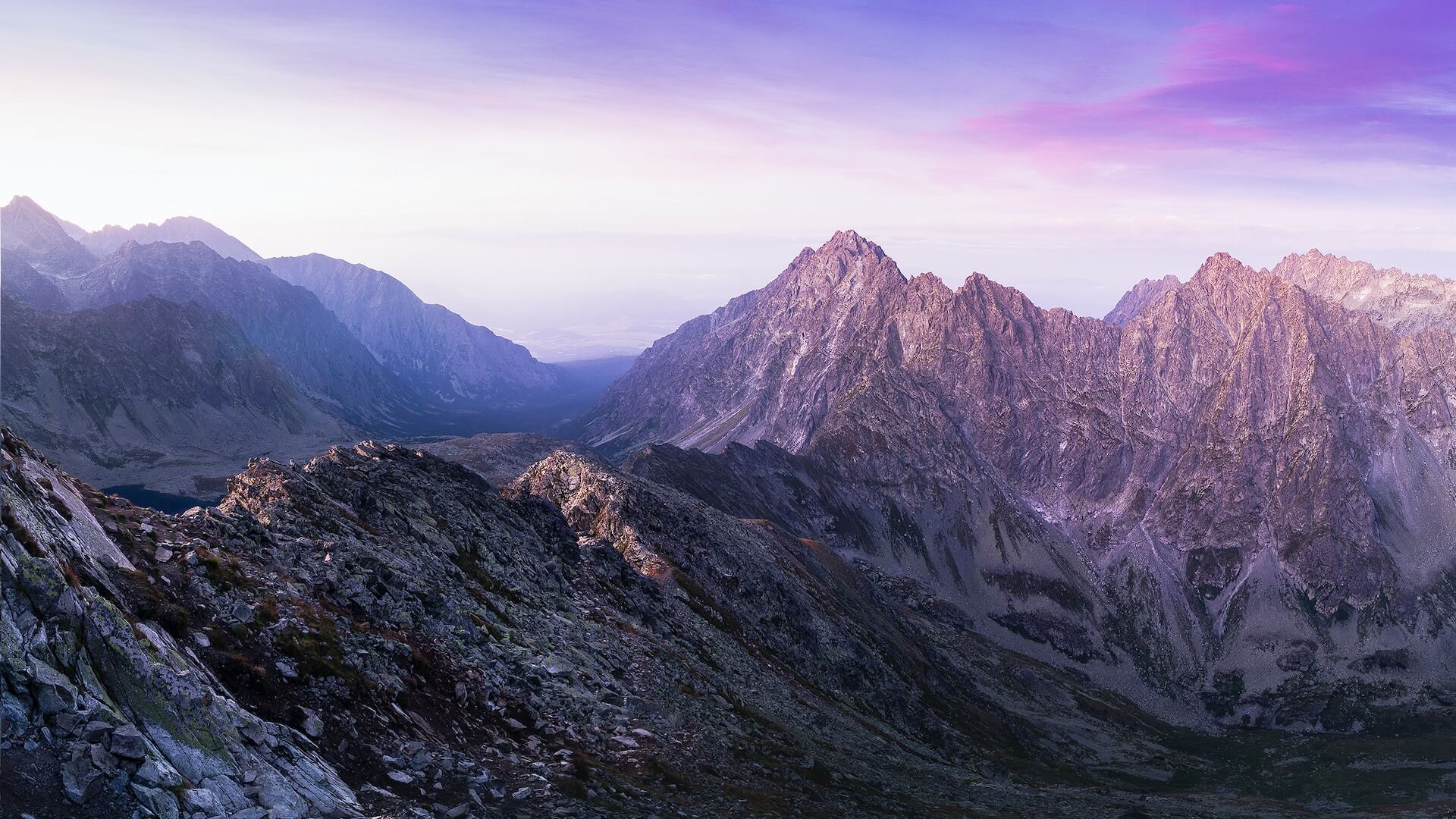
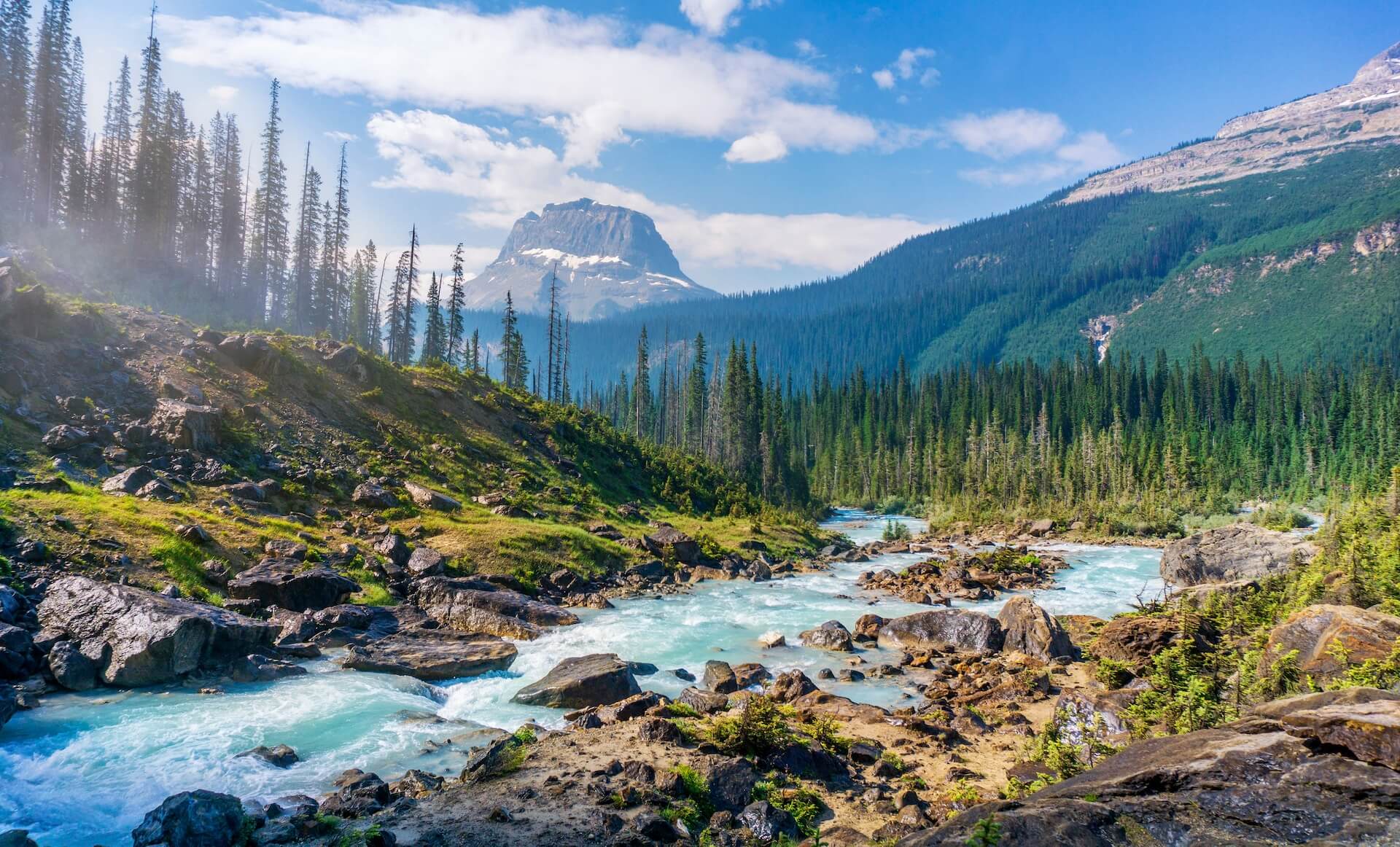
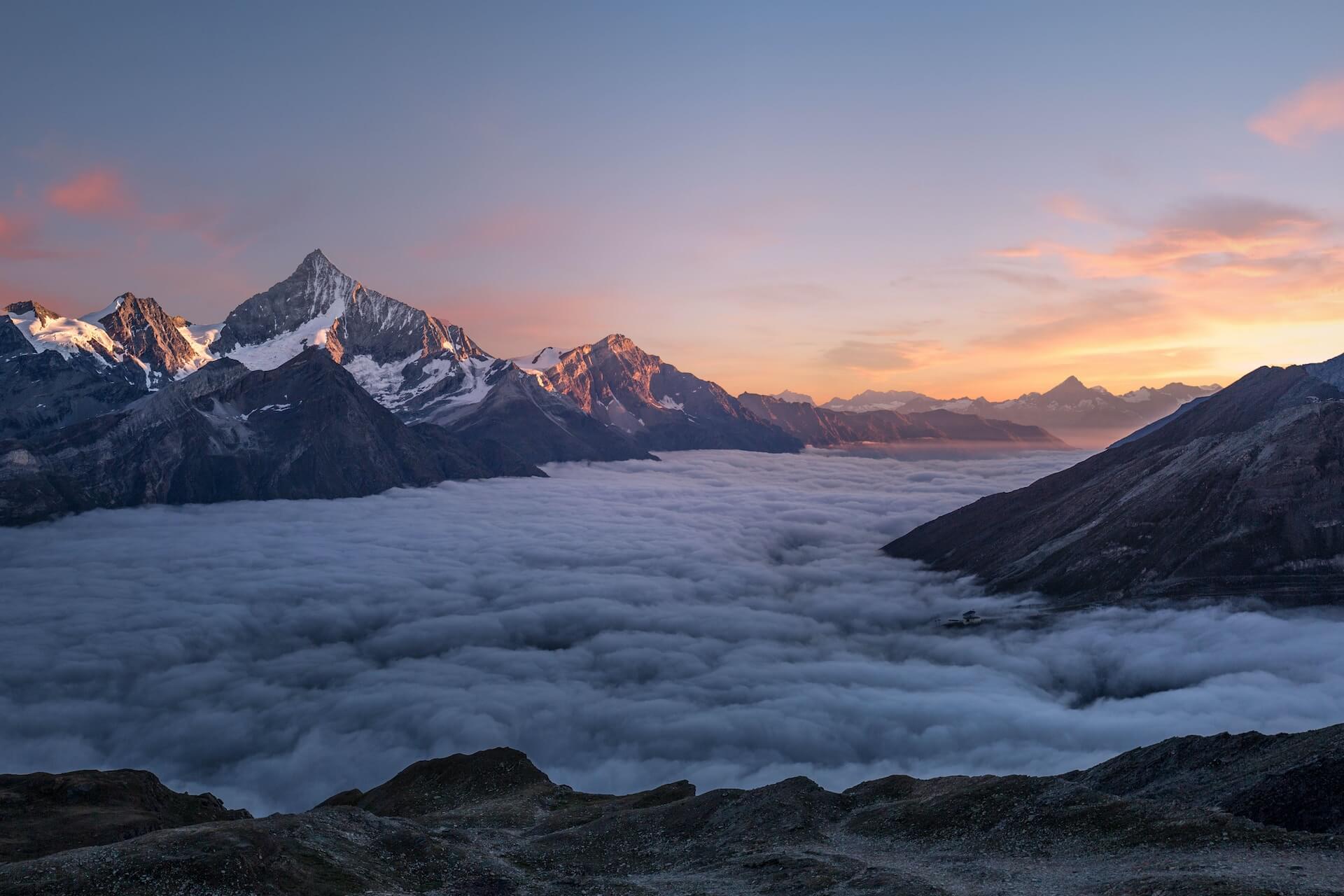
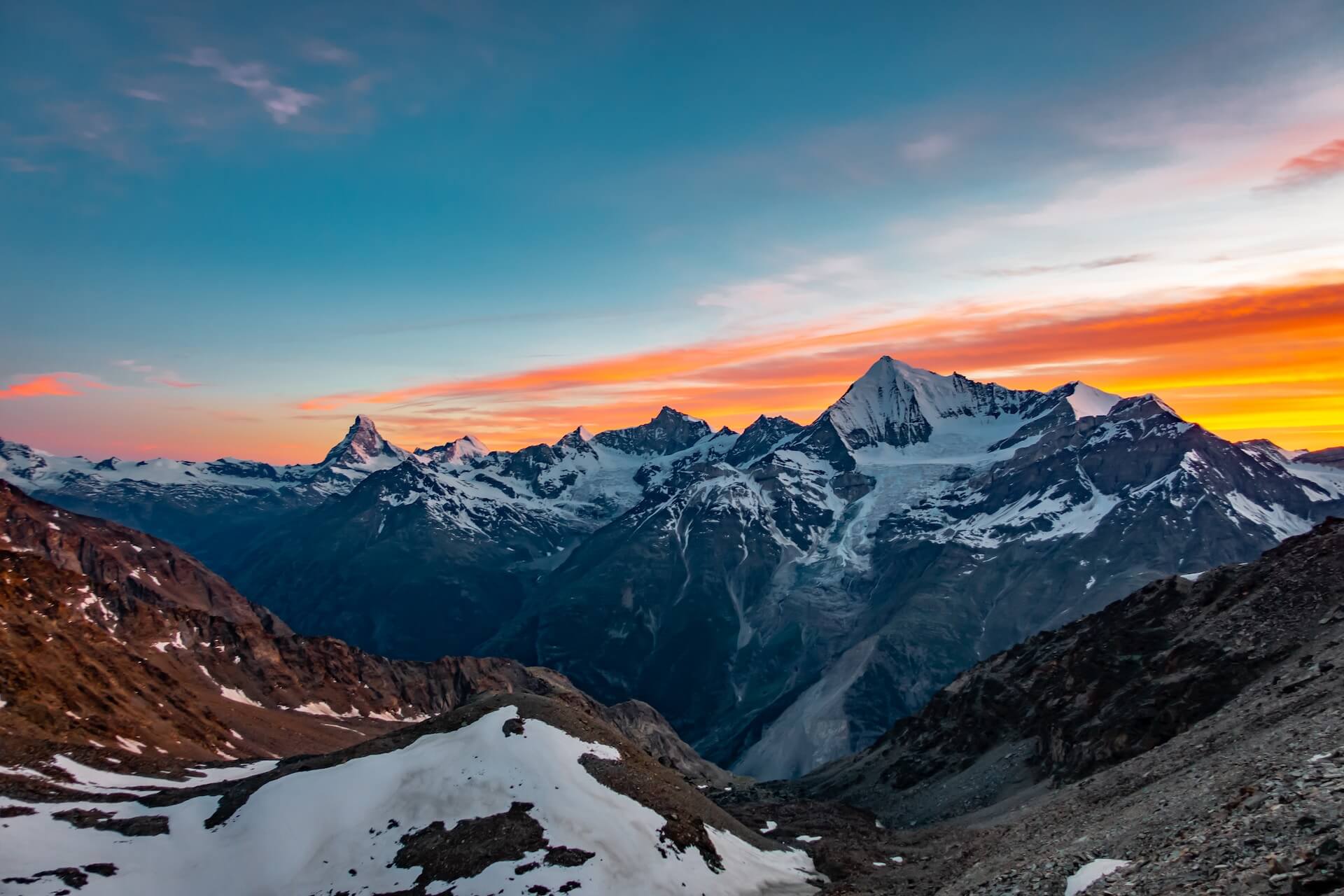
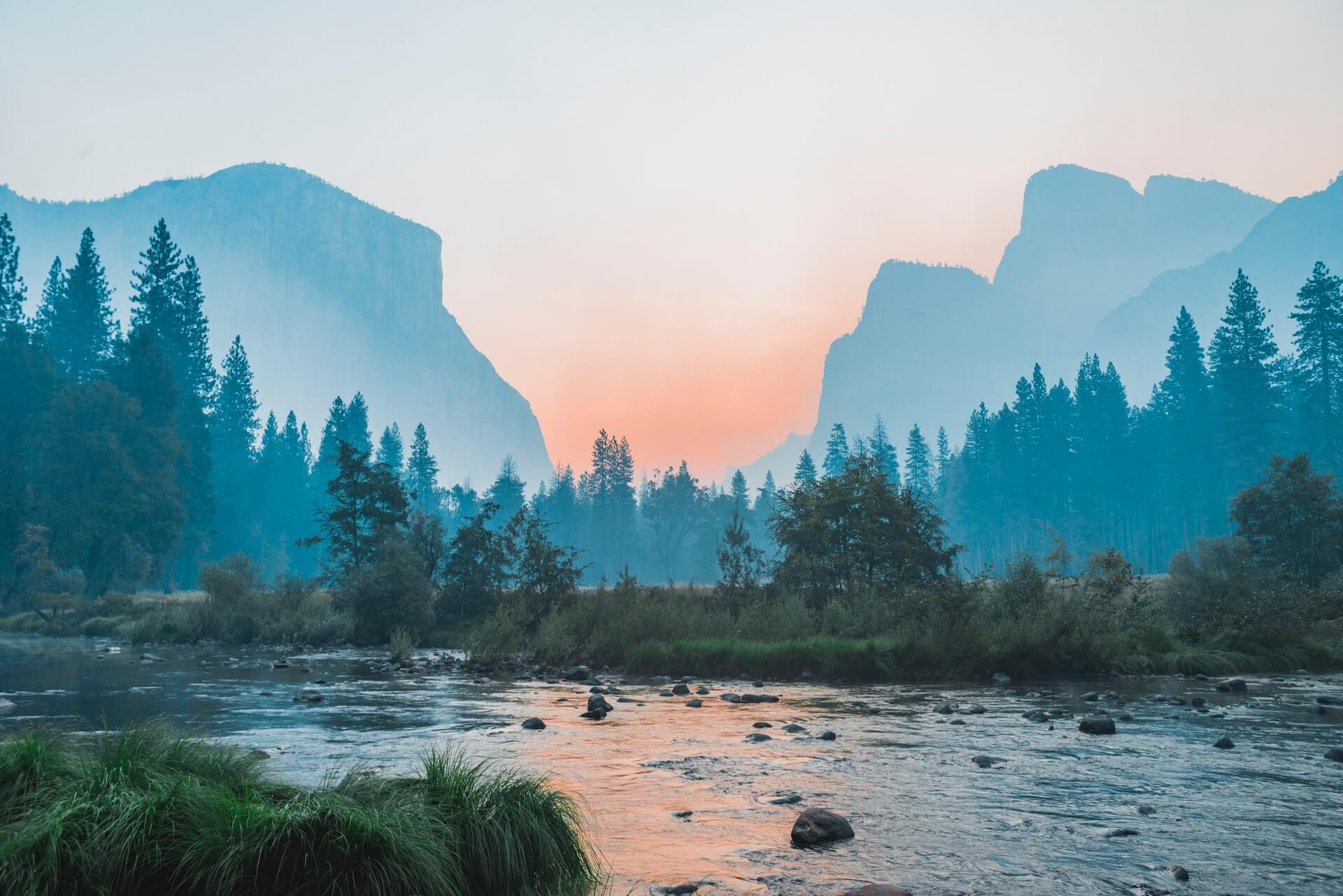
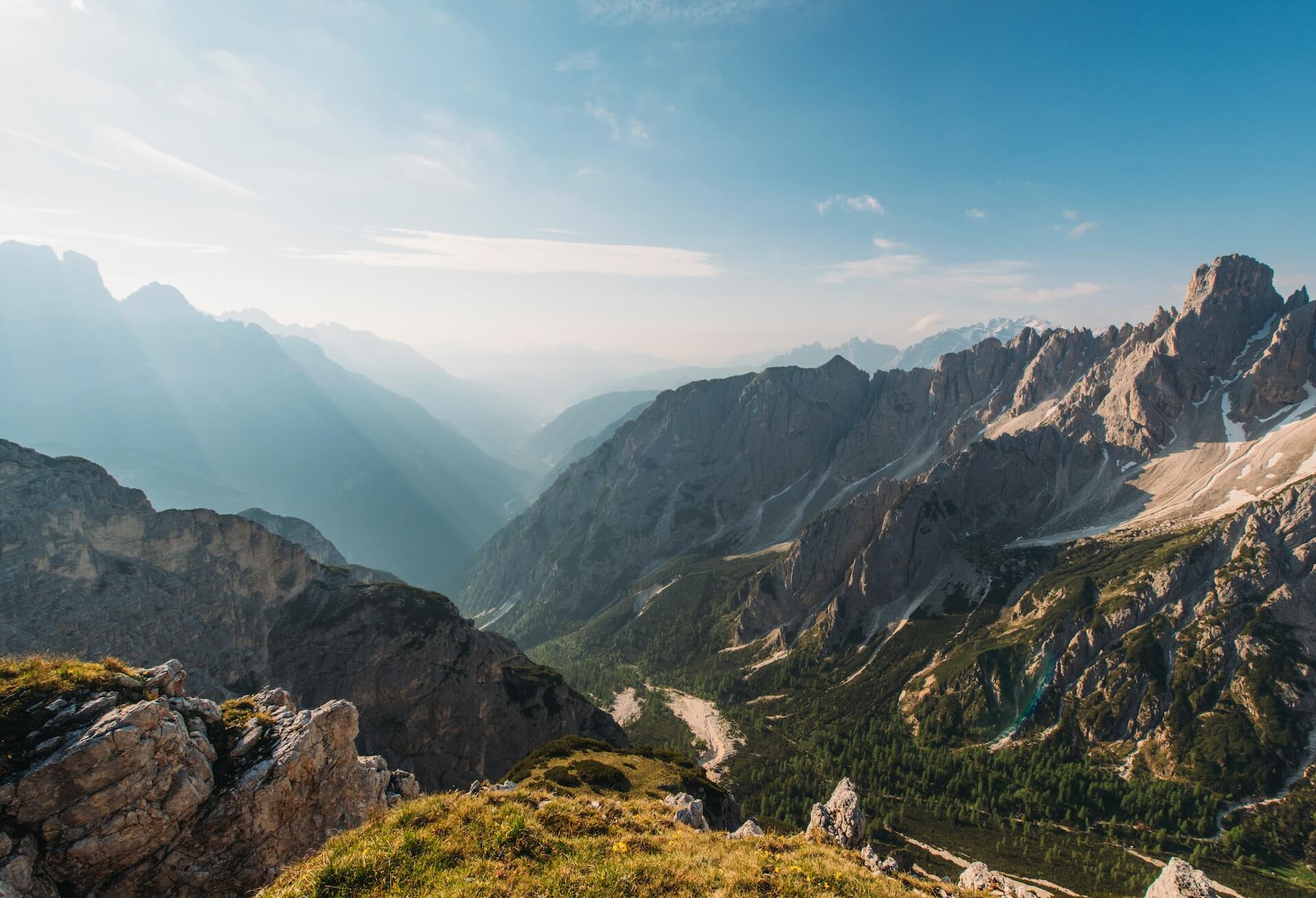
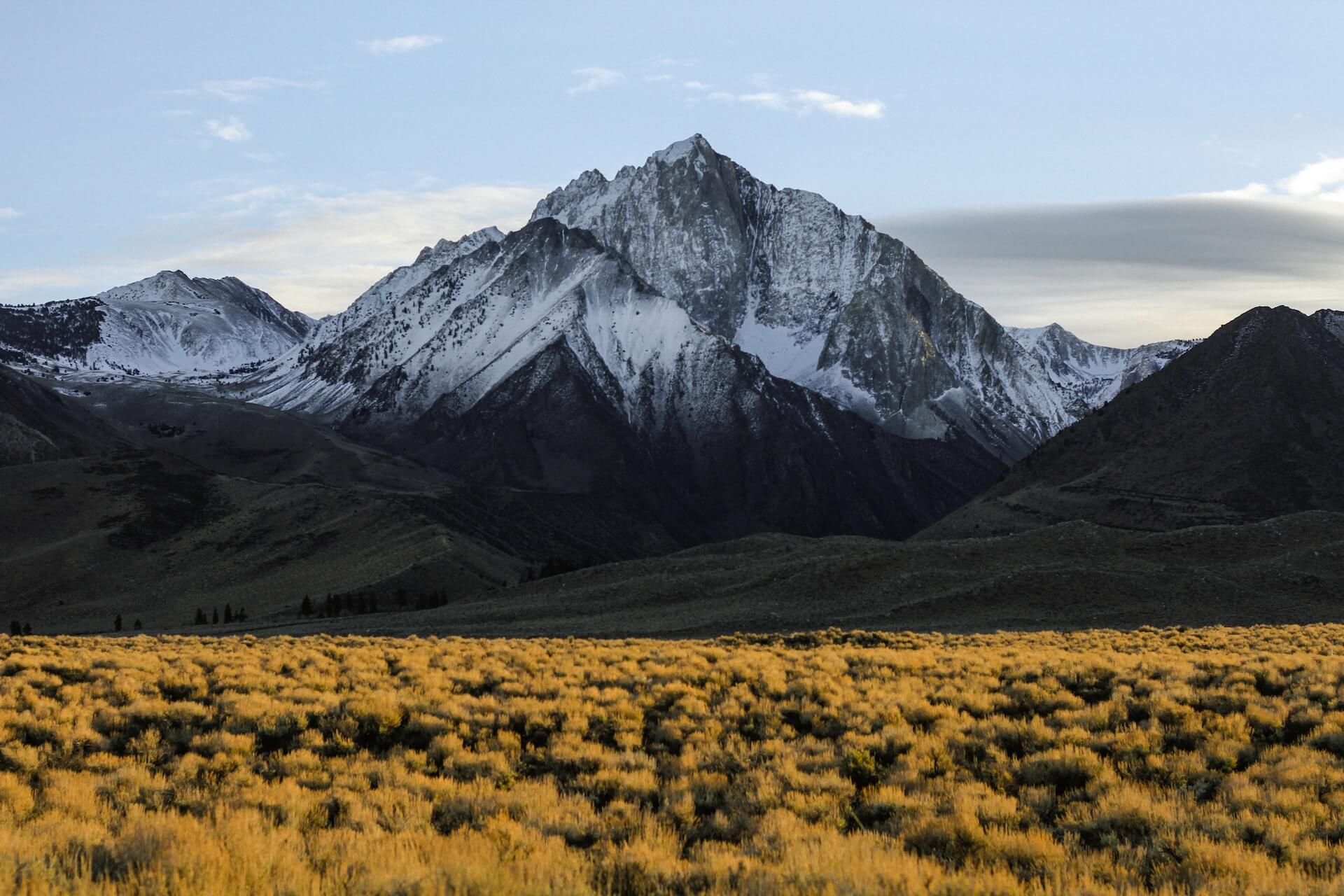
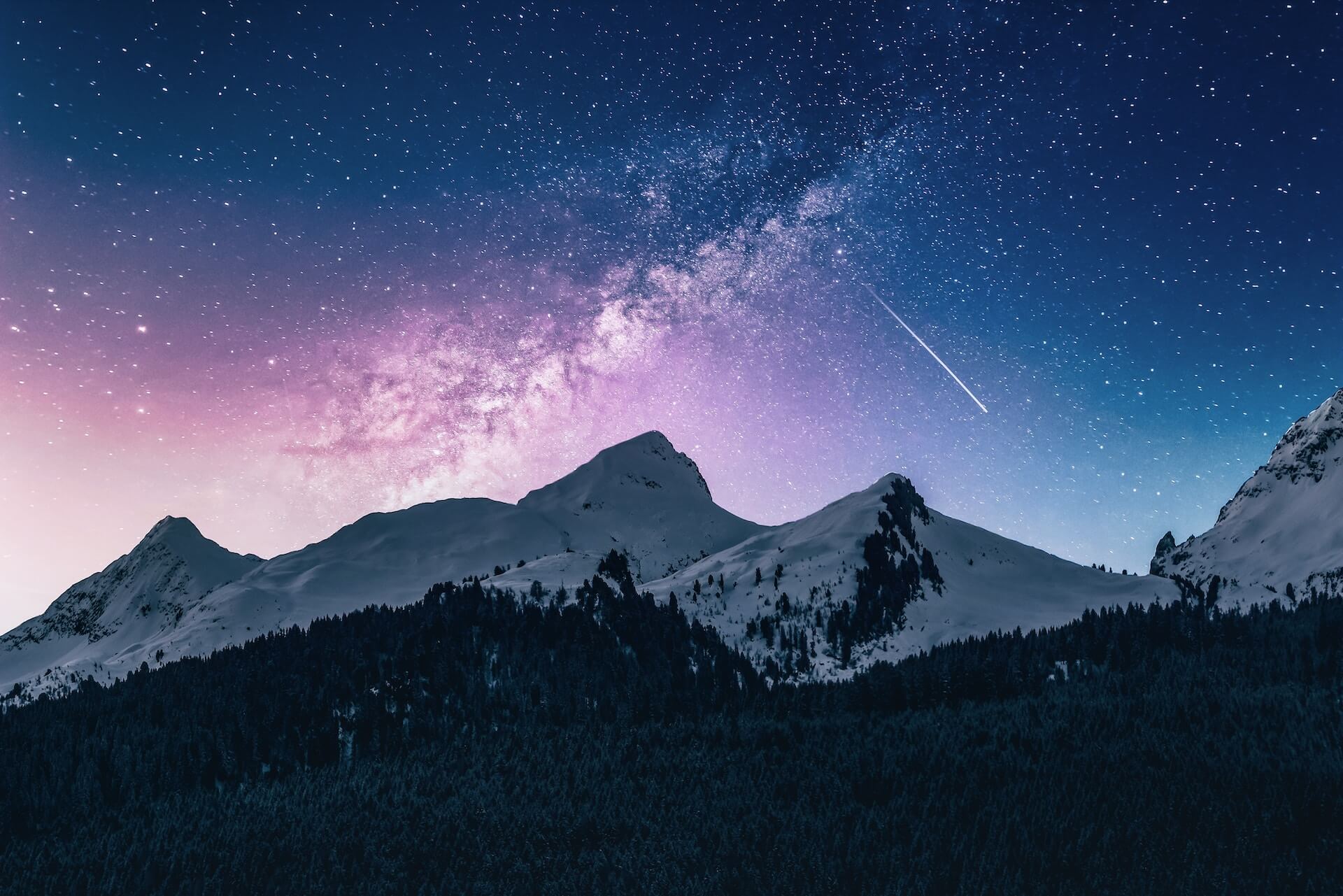
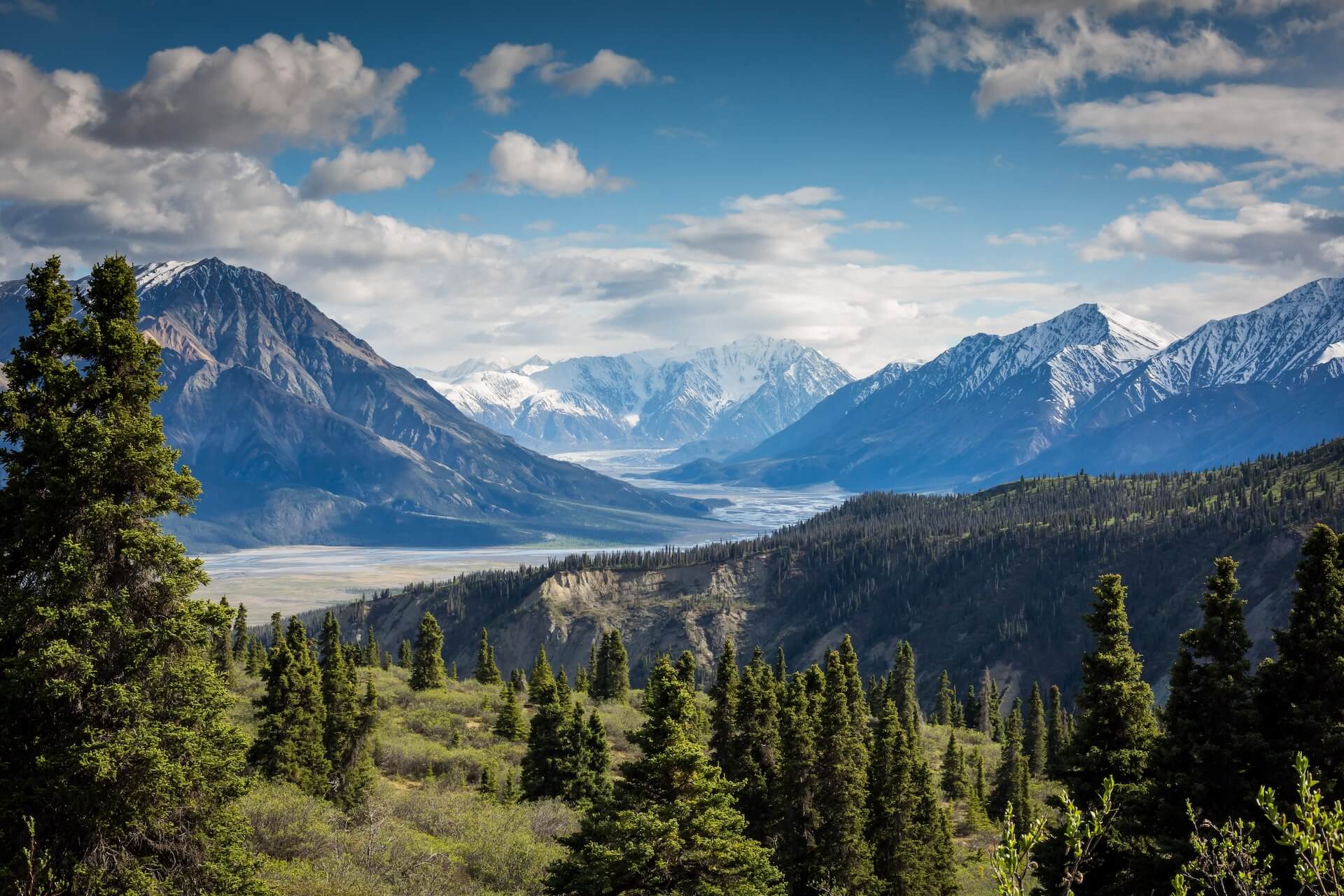
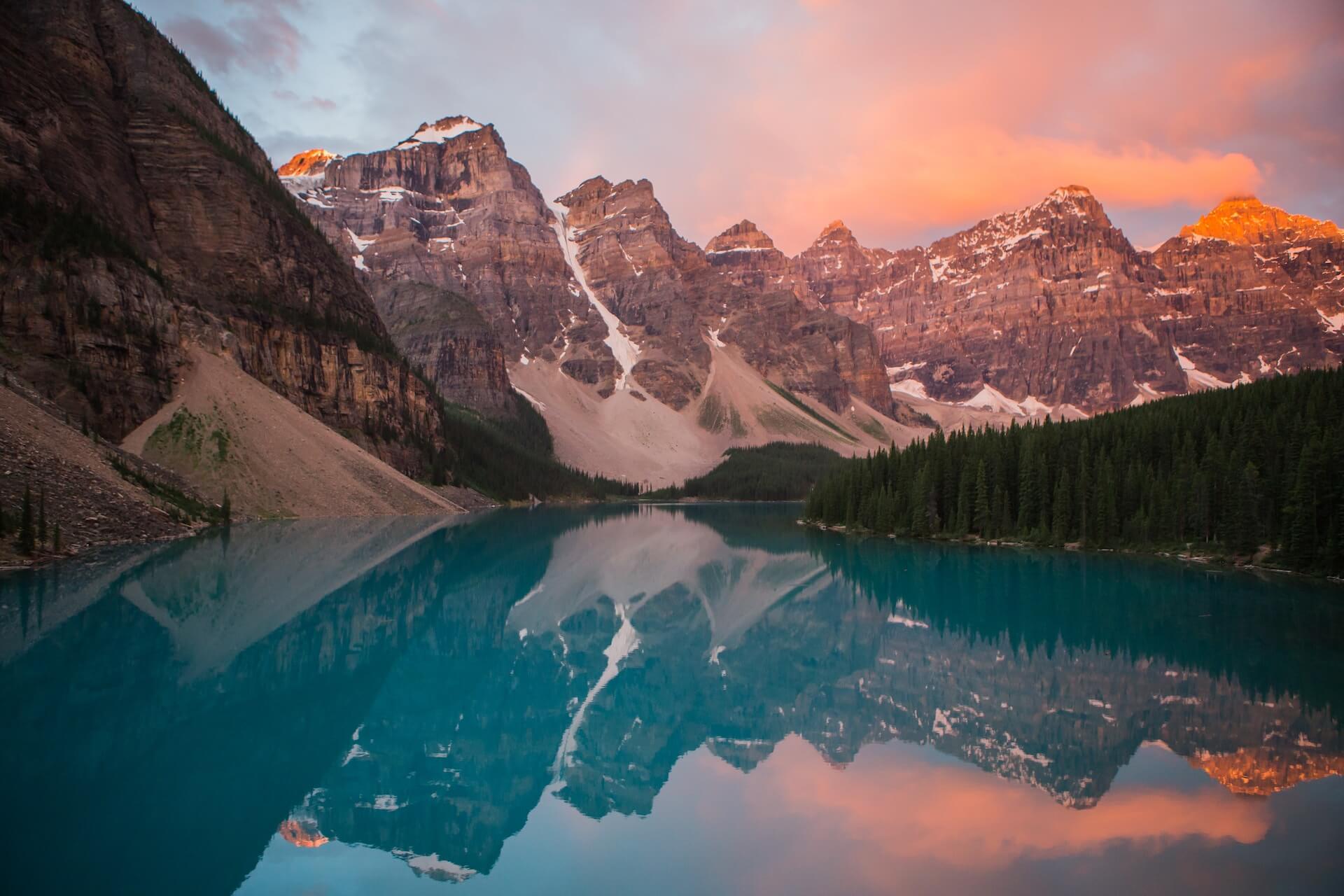
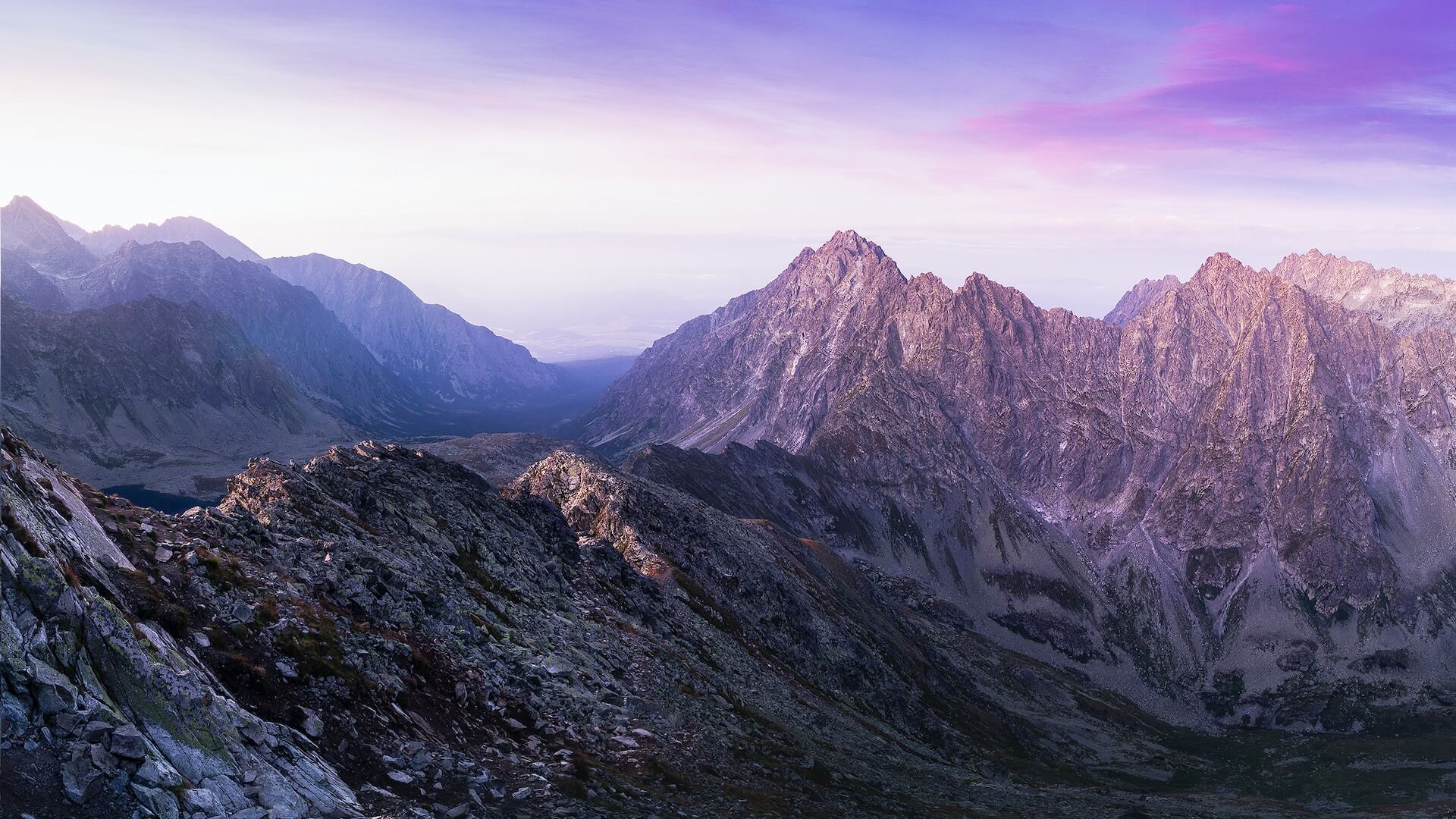
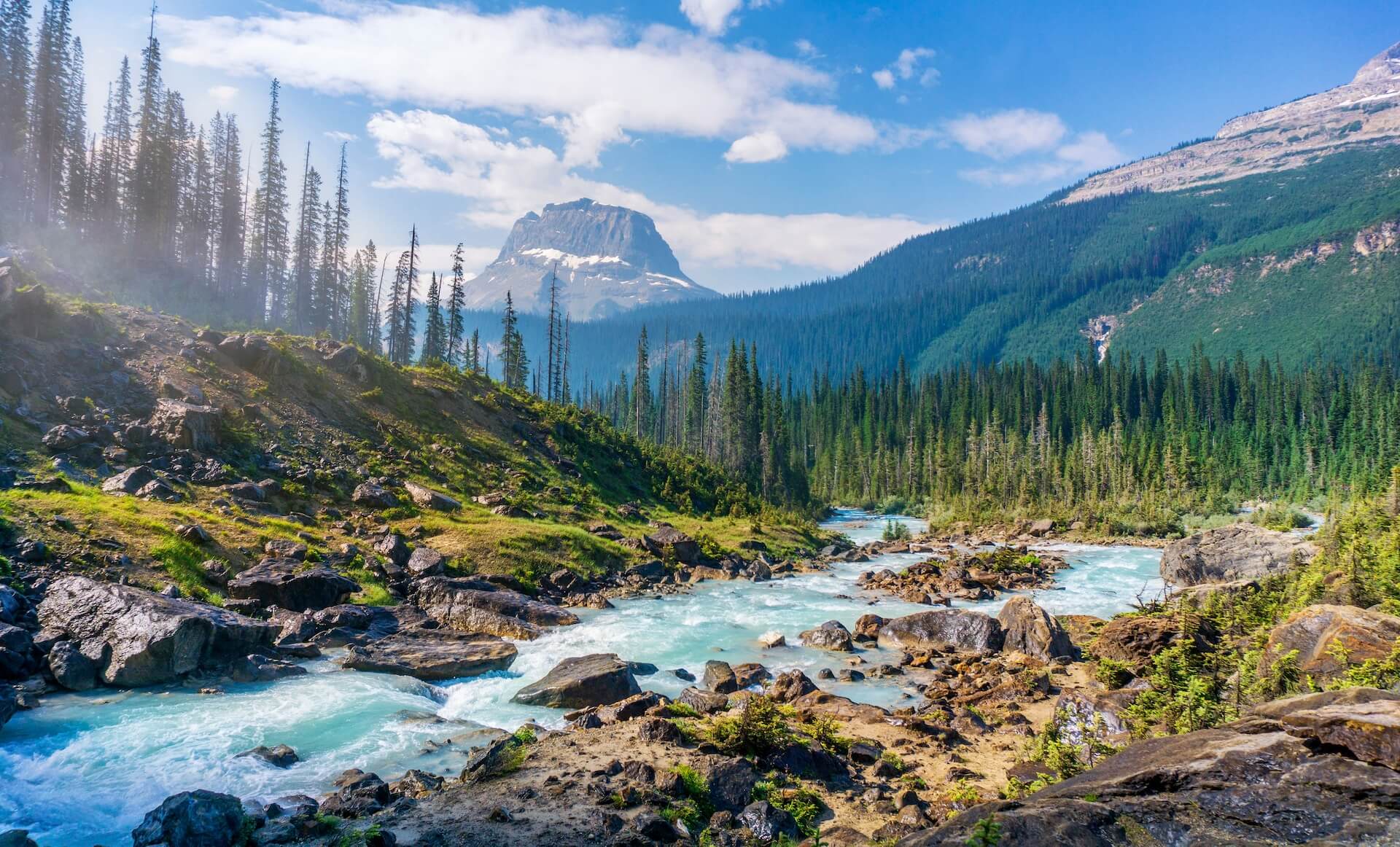
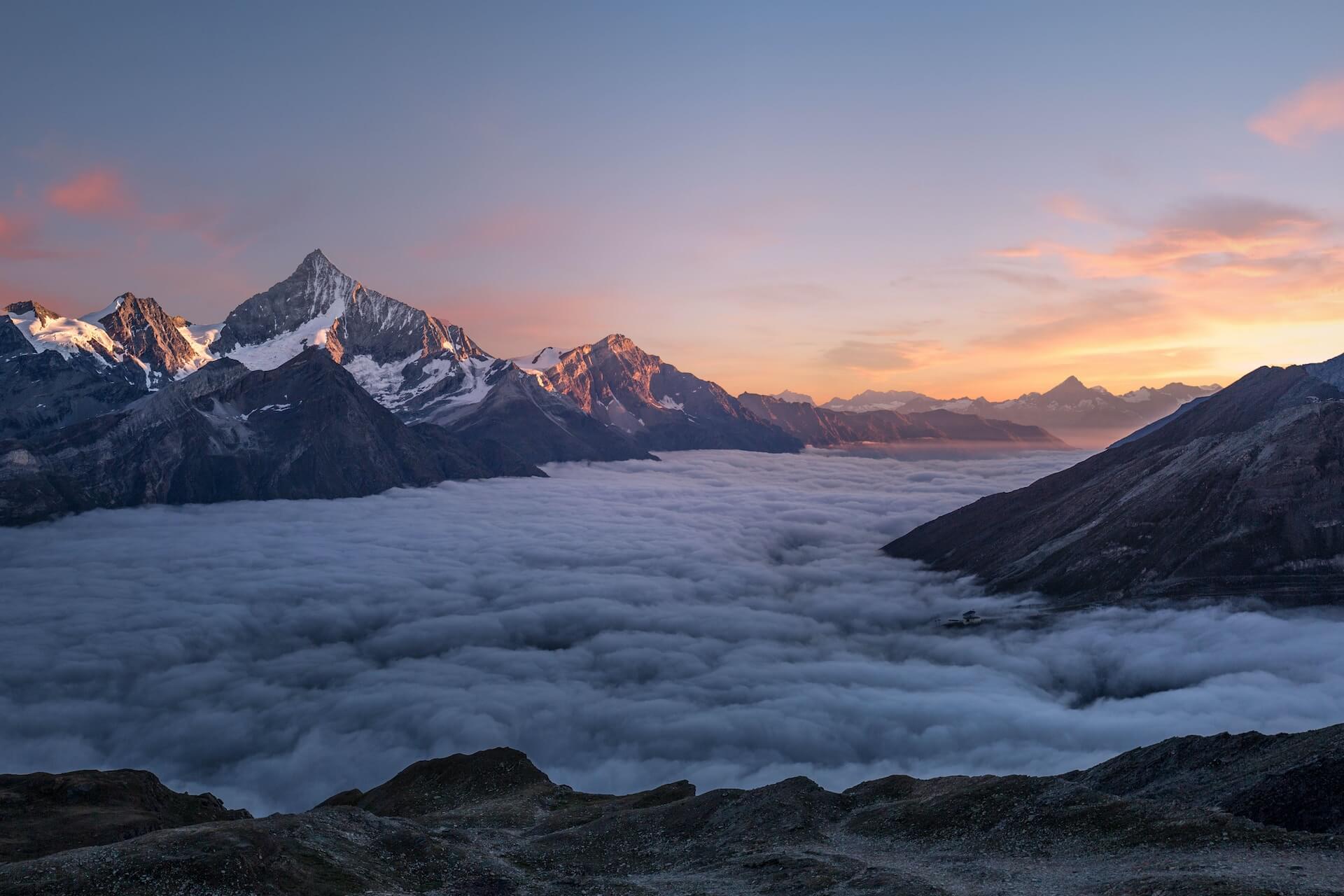
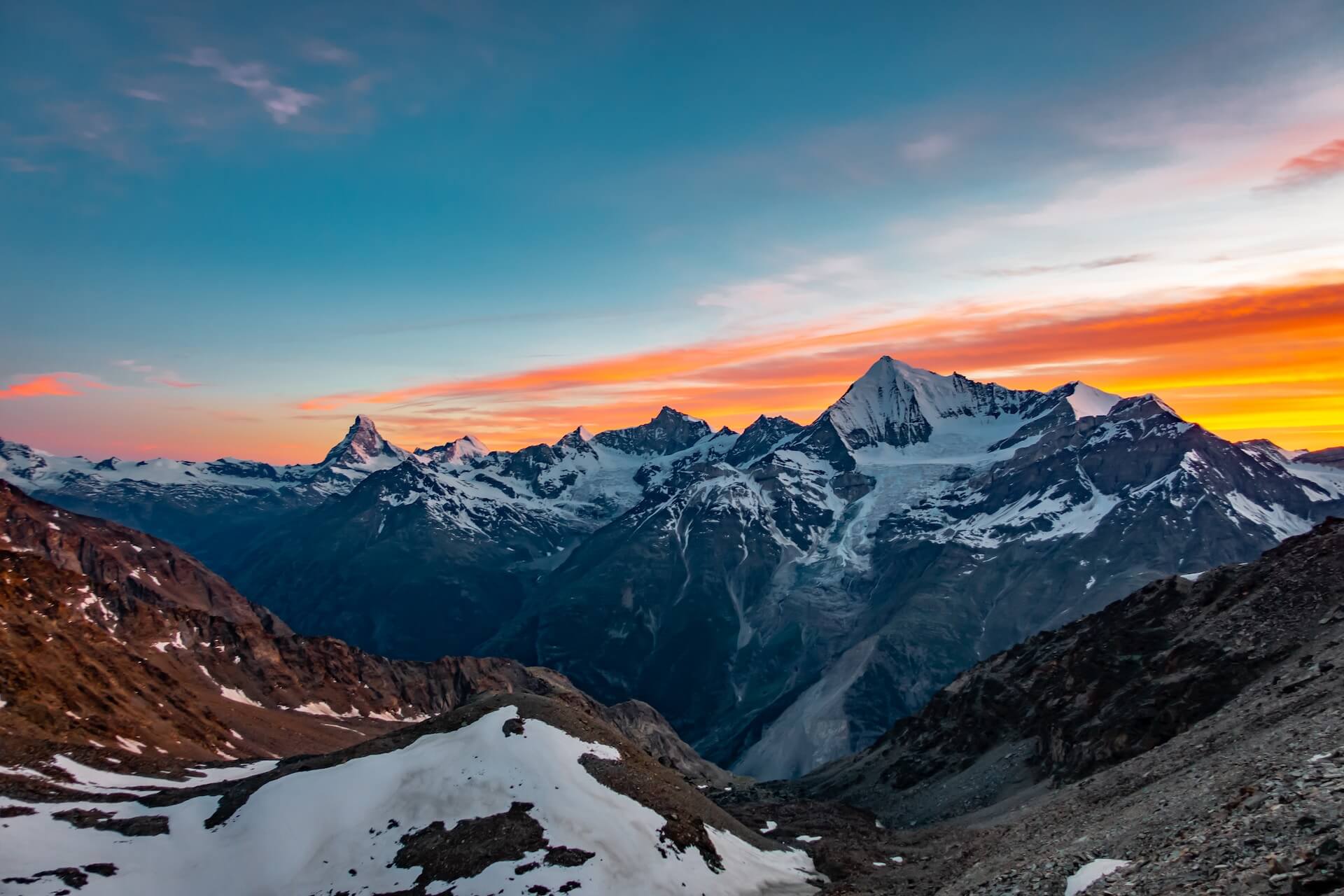
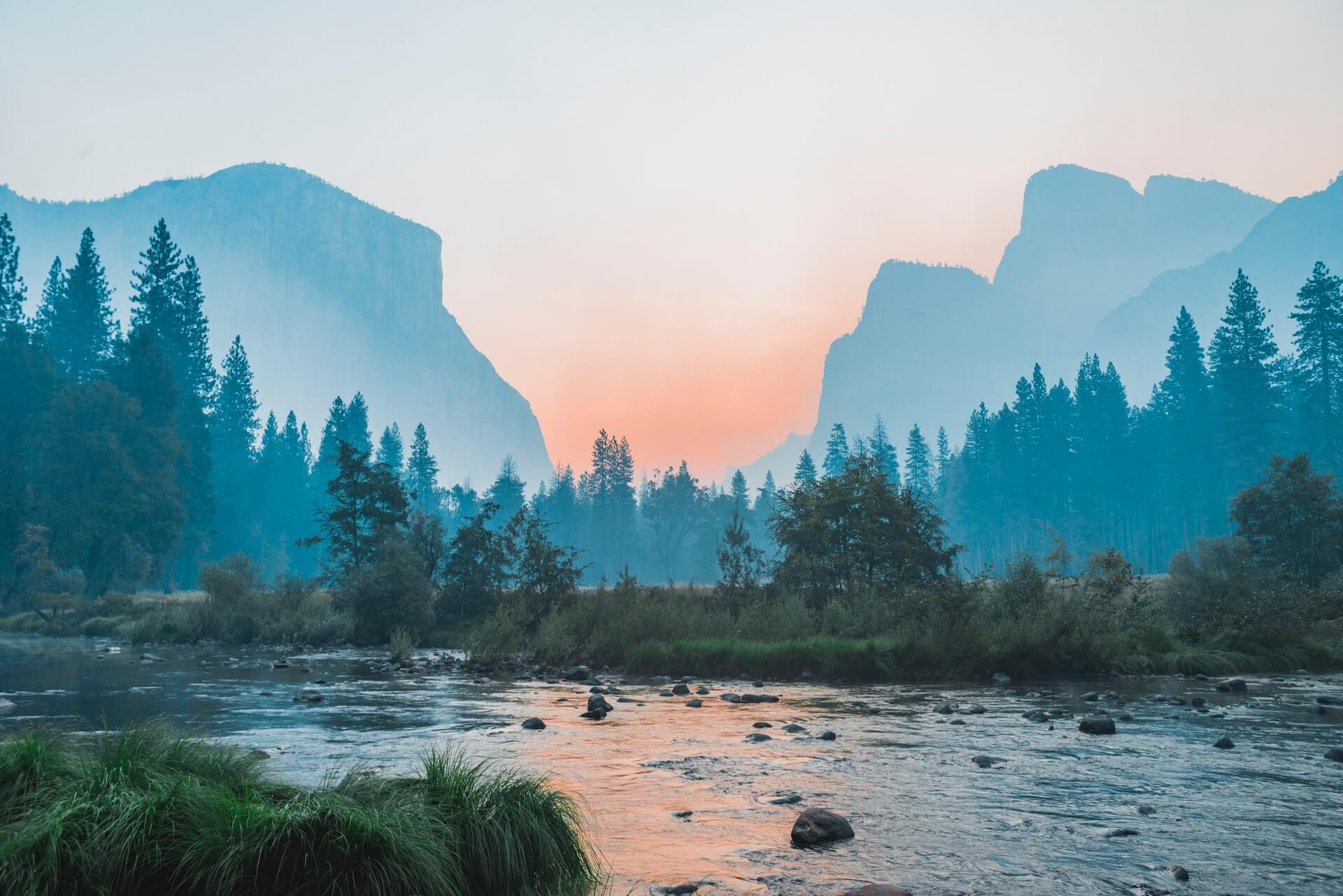
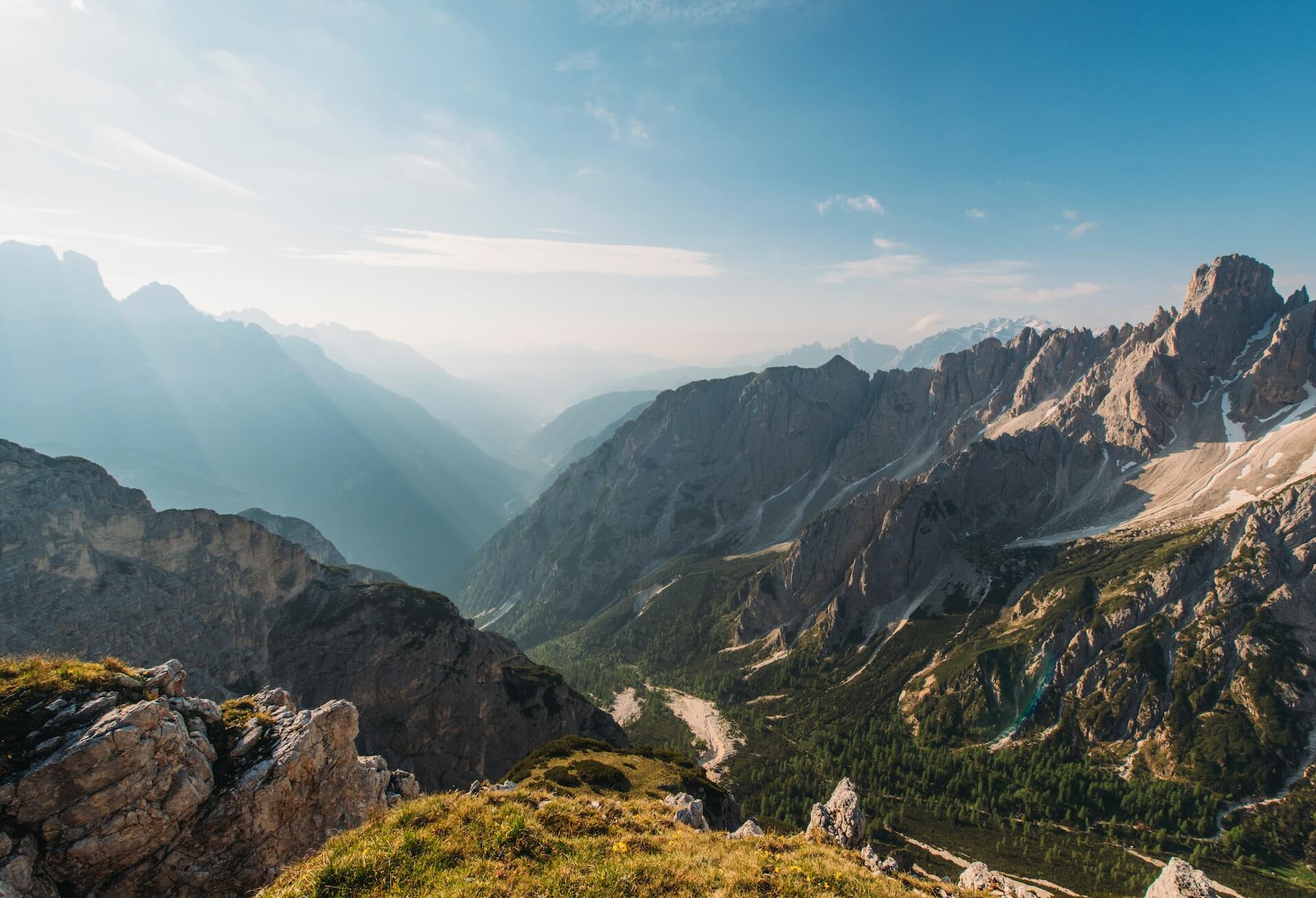
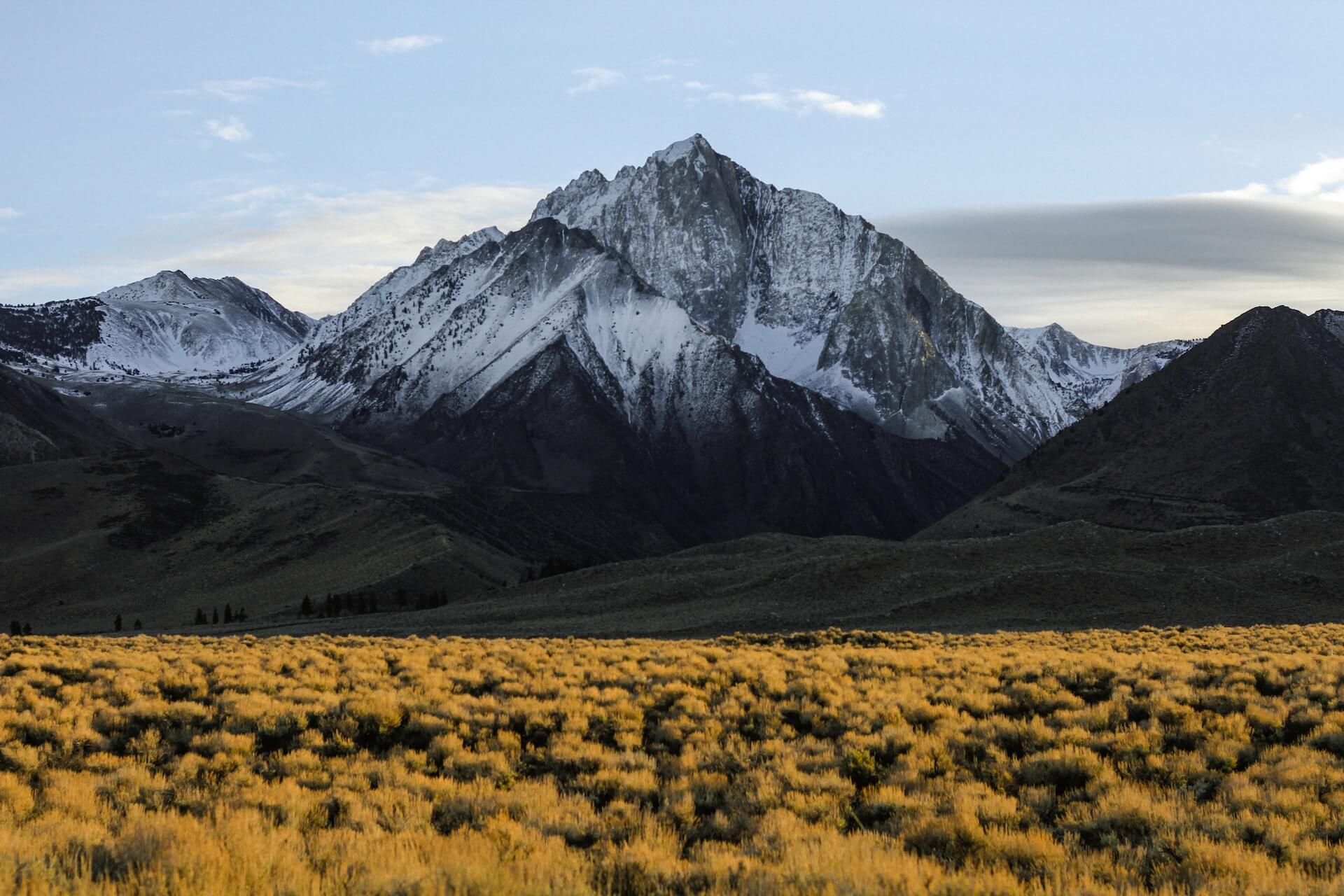
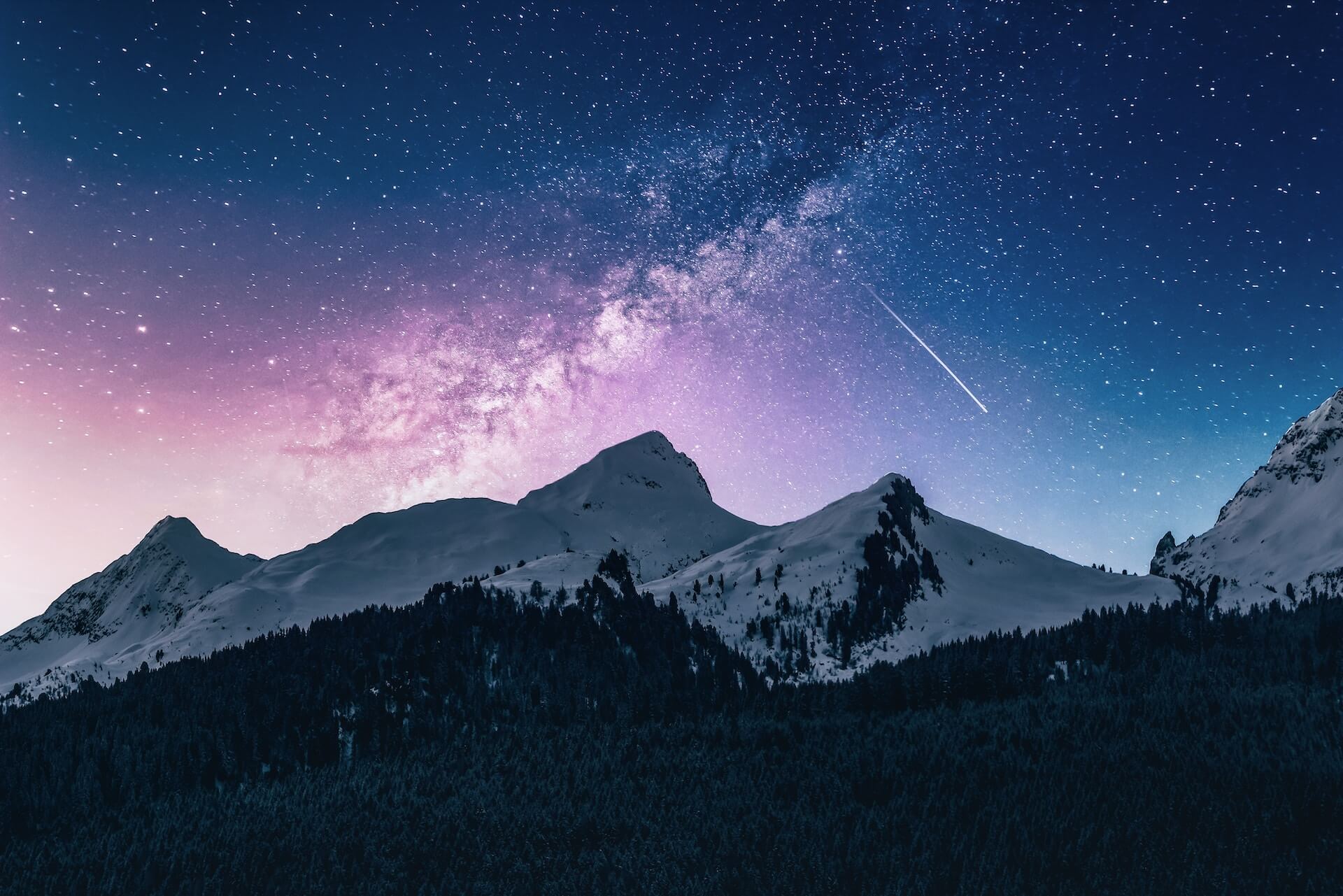
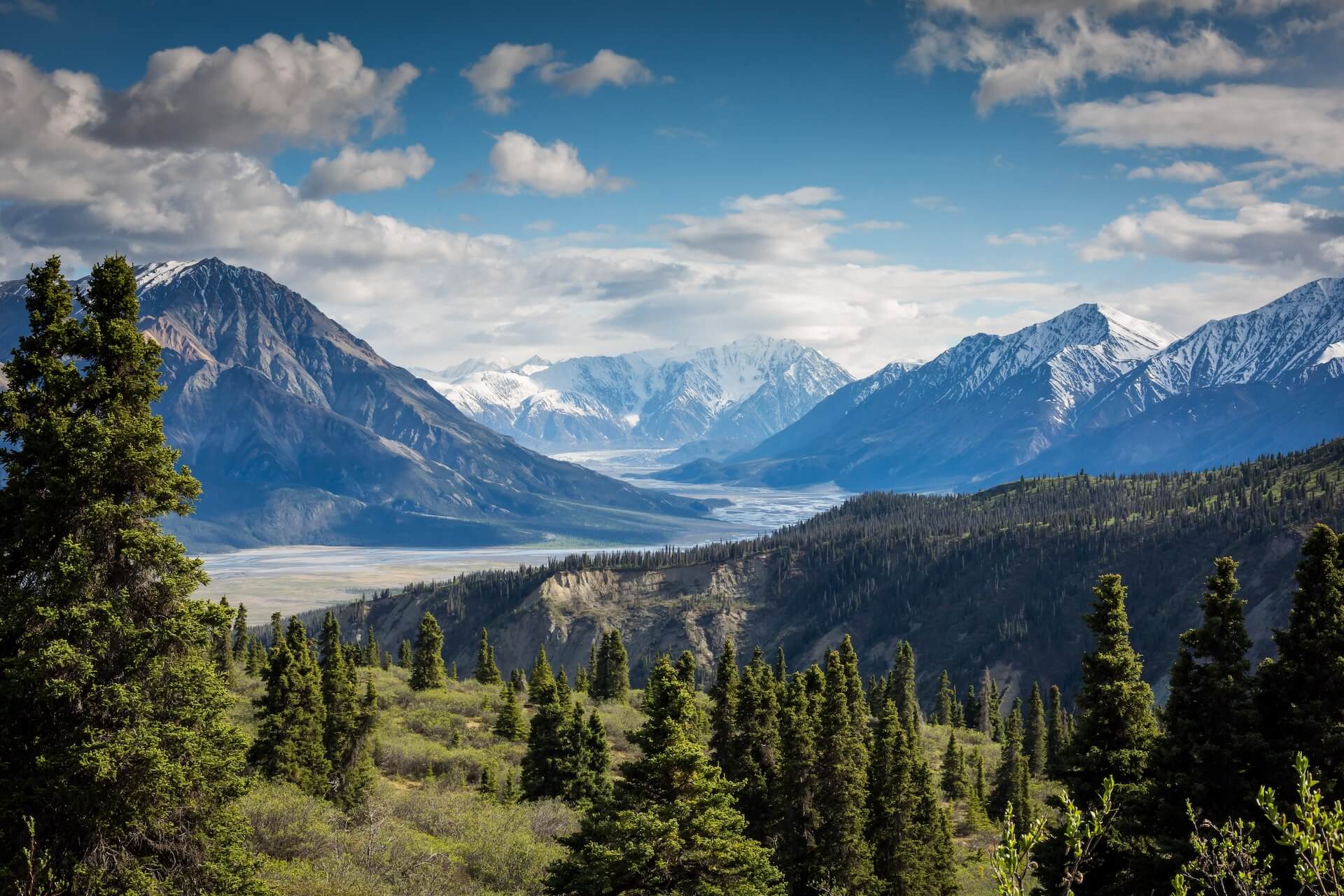
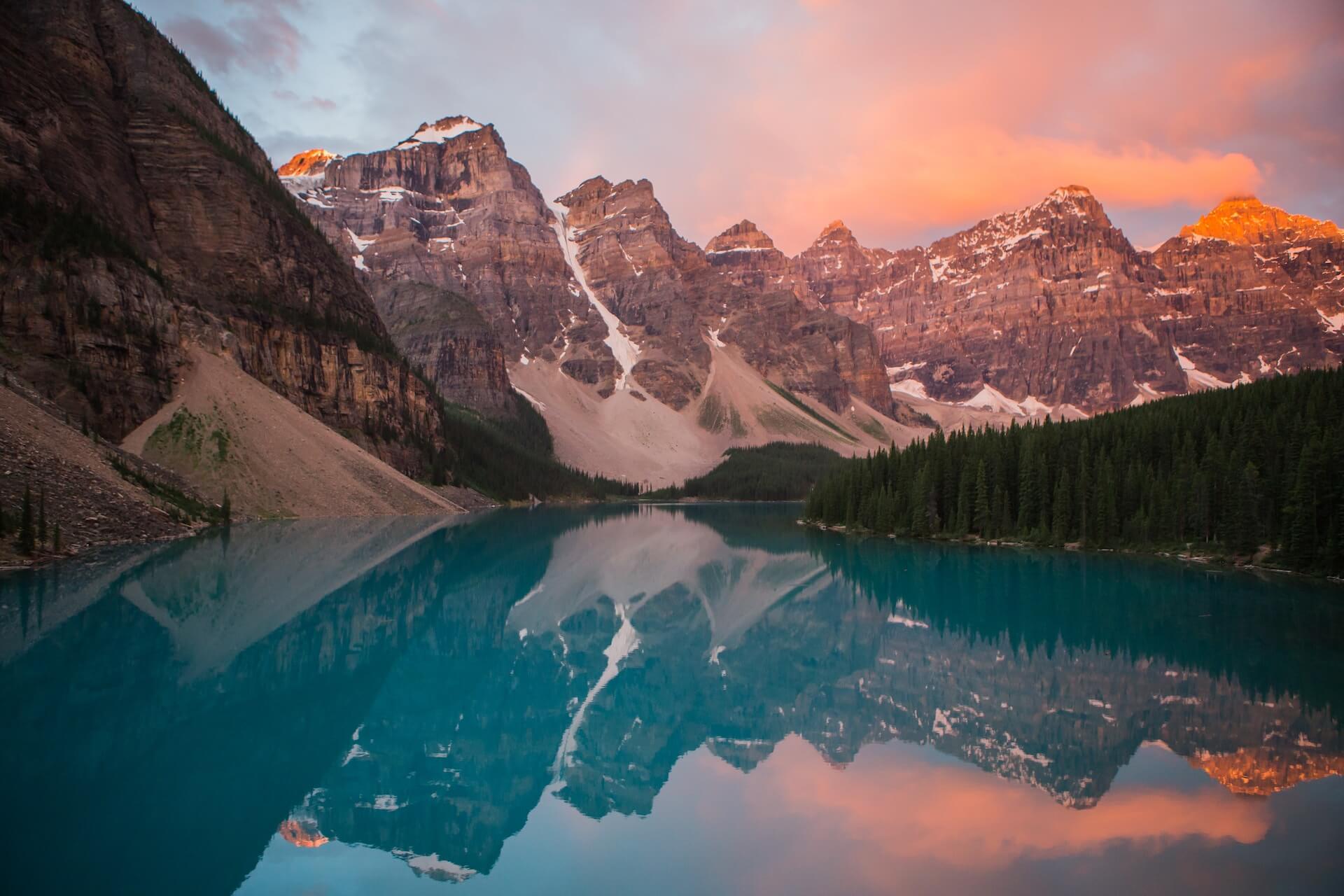
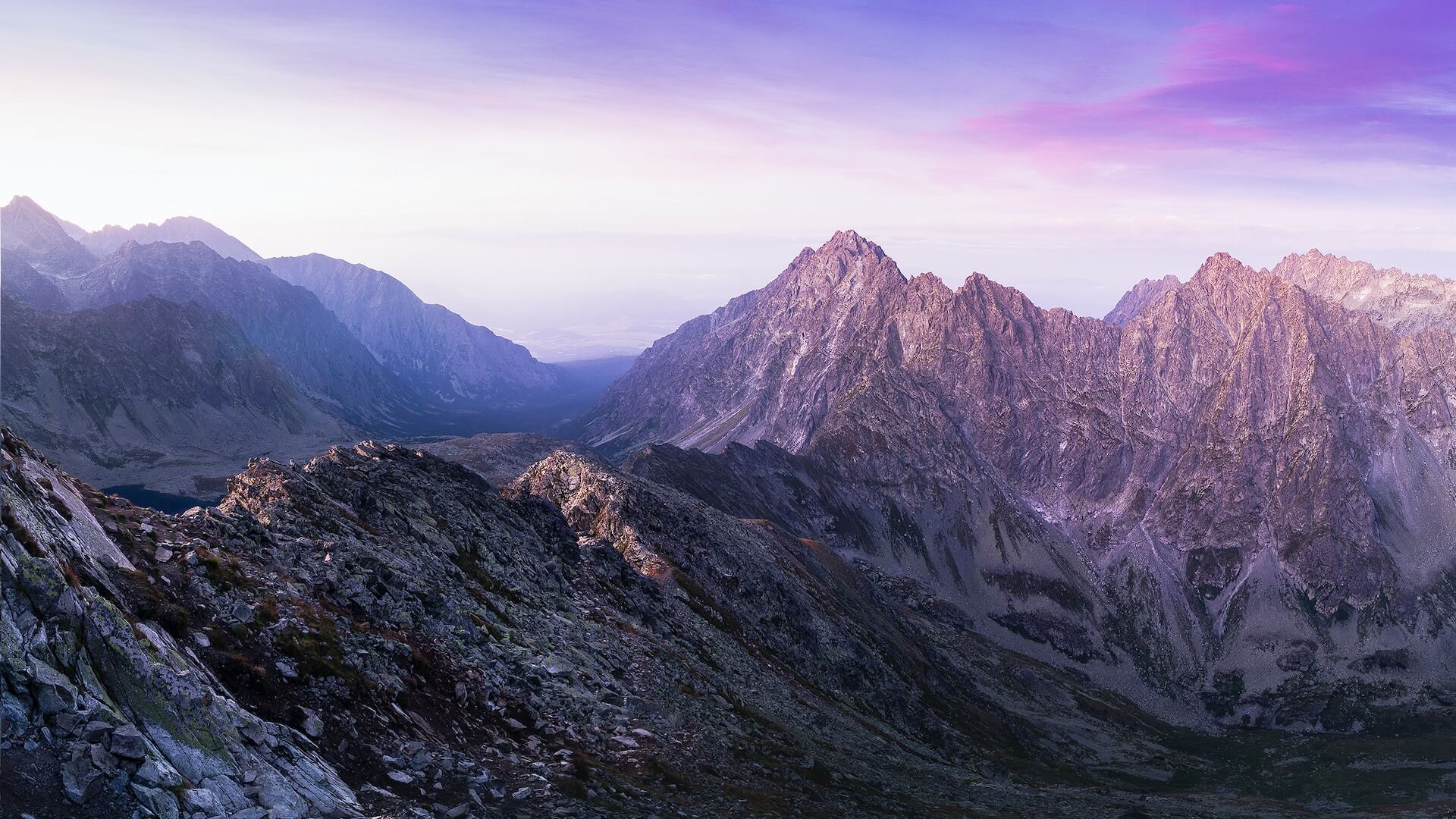
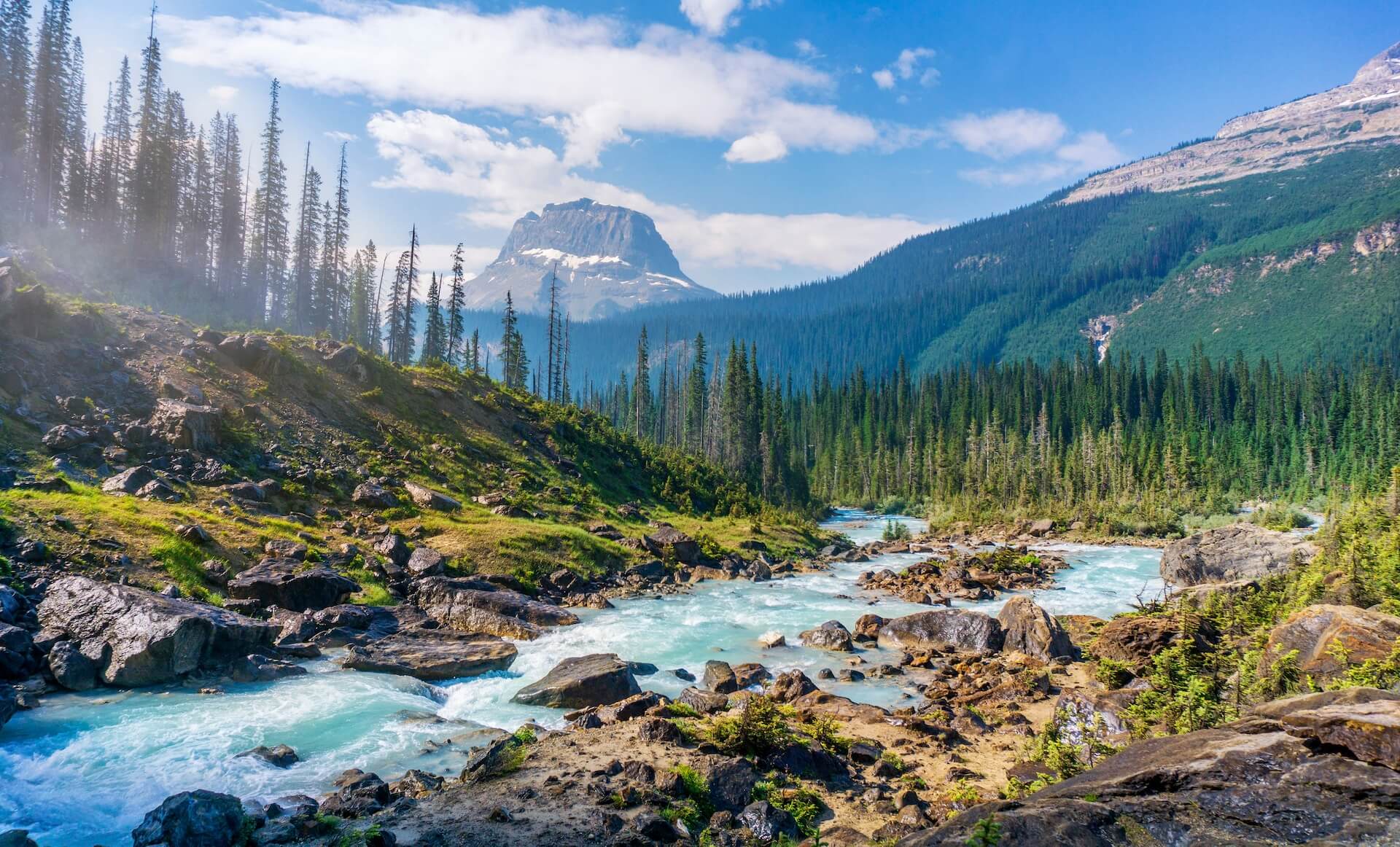
Copied !
---
import CarouselGallery from "$components/gallery/carousel/CarouselGallery.astro";
import type { ImageType } from "$common/types";
const images: ImageType[] = [
{
src: "https://cdn.devdojo.com/images/june2023/mountains-01.jpeg",
alt: "Photo of Mountains",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-02.jpeg",
alt: "Photo of Mountains 02",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-03.jpeg",
alt: "Photo of Mountains 03",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-04.jpeg",
alt: "Photo of Mountains 04",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-05.jpeg",
alt: "Photo of Mountains 05",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-06.jpeg",
alt: "Photo of Mountains 06",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-07.jpeg",
alt: "Photo of Mountains 07",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-08.jpeg",
alt: "Photo of Mountains 08",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-09.jpeg",
alt: "Photo of Mountains 09",
},
{
src: "https://cdn.devdojo.com/images/june2023/mountains-10.jpeg",
alt: "Photo of Mountains 10",
},
];
---
<div class="bg-slate-200 p-4 flex flex-col gap-y-8 relative w-full">
<CarouselGallery {images} />
<CarouselGallery direction="vertical" {images} />
<CarouselGallery thumbnailWidth="200px" {images} />
</div>